Introduction
Unlocking the potential for intuitive and expressive code, operator overloading in Python stands as a cornerstone of flexibility and customizability. It empowers builders to infuse their courses with operator semantics, bridging the hole between summary ideas and concrete implementations. By reimagining operators reminiscent of +, -, *, or inside customized courses, Python transcends standard programming norms, fostering concise and readable code paying homage to mathematical expressions. This text units forth on an expedition by means of the universe of operator overloading, illuminating its intricacies, advantages, and sensible functions spanning many domains in Python programming.
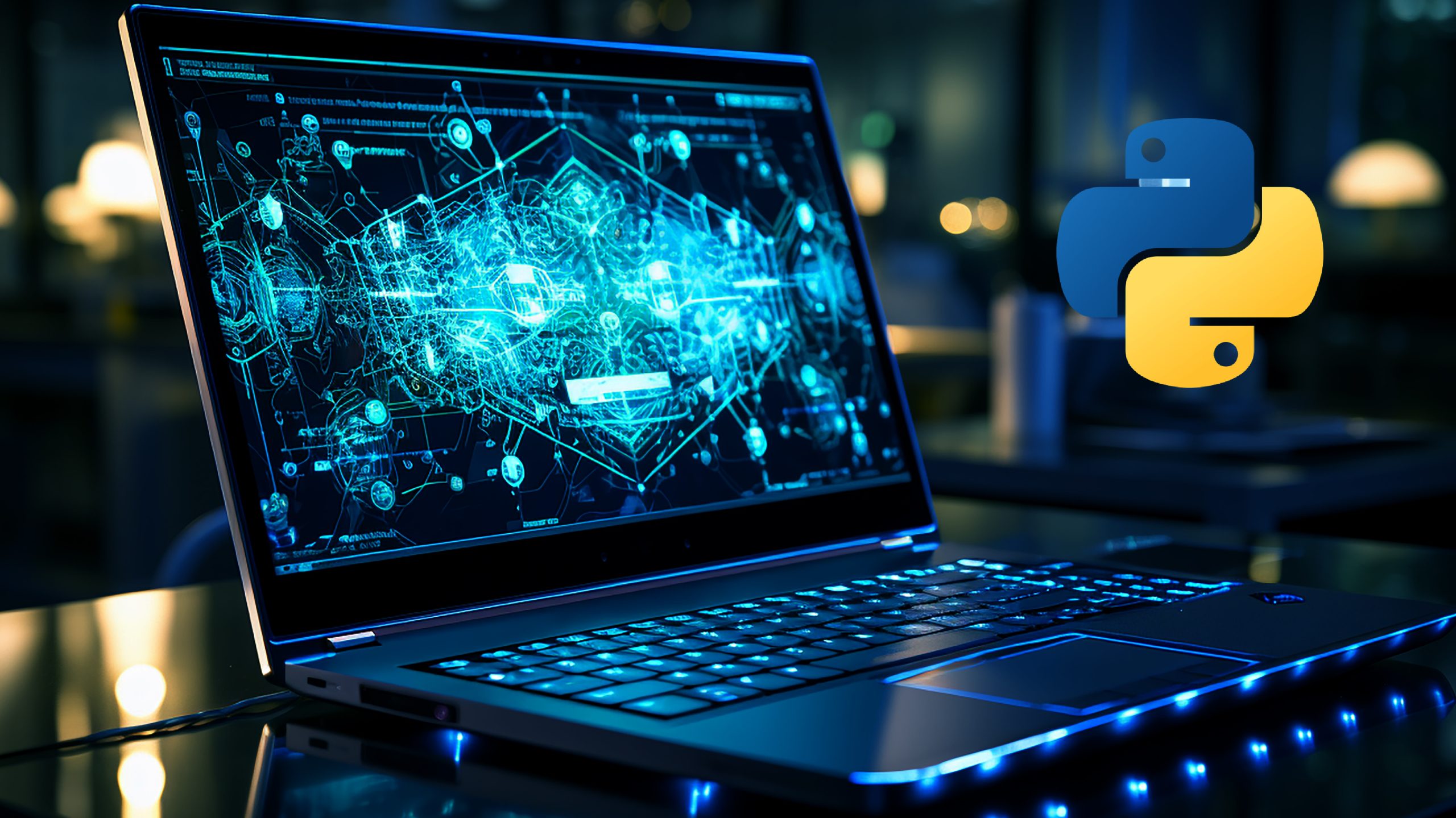
Understanding Operator Overloading
Once we carry out operations on objects of a category utilizing operators, Python seems for particular strategies within the class definition that correspond to these operators. For instance, once we use the + operator, Python seems for the __add__() technique within the class definition.
Let’s take an instance to know this higher. Suppose we’ve a category known as Level that represents a degree in 2D area. On this class, we are able to outline the __add__() technique so as to add two Level objects collectively.
Code
class Level:
    def __init__(self, x, y):
        self.x = x
        self.y = y
    def __add__(self, different):
        return Level(self.x + different.x, self.y + different.y)
p1 = Level(1, 2)
p2 = Level(3, 4)
consequence = p1 + p2
print(consequence.x, consequence.y)
Output
4 6
Advantages of Operator Overloading in Python
- Enhanced Readability: Operator overloading enhances code readability and intuitiveness. Quite than invoking strategies like add(), the + operator instantly streamlines comprehension.
- Conciseness: Overloading operators allows the creation of succinct code that carefully mirrors mathematical expressions, fostering brevity in implementation.
- Tailor-made Customization: The characteristic permits customization of operator behaviors tailor-made to particular courses, granting builders granular management over object-operator interactions.
- Versatile Flexibility: Operator overloading empowers the definition of operators for customized courses not inherently current in Python, facilitating the creation of specialised operations aligned with particular domains.
- Seamless Compatibility: By embracing operator overloading, customized objects harmonize with present Python code using built-in operators, making certain clean integration inside broader codebases.
Additionally learn: 15 Finest Python Books For You
Implementing Operator Overloading in Python
Listed here are the methods to implement operator overloading in Python:
Arithmetic Operators
Arithmetic operators like `+,` `-,` `*,` and `/` will be overloaded in Python. Let’s see an instance:
Code
class Level:
    def __init__(self, x, y):
        self.x = x
        self.y = y
    def __add__(self, different):
        return Level(self.x + different.x, self.y + different.y)
p1 = Level(1, 2)
p2 = Level(3, 4)
consequence = p1 + p2
print(consequence.x, consequence.y)
Output
4 6
Comparability Operators
Comparability operators like `==`, `!=`, `<`, `>`, `<=`, `>=` will also be overloaded. Right here’s an instance:
Code
class Level:
    def __init__(self, x, y):
        self.x = x
        self.y = y
    def __eq__(self, different):
        return self.x == different.x and self.y == different.y
p1 = Level(1, 2)
p2 = Level(1, 2)
print(p1 == p2)
Output
True
Project Operators
Project operators like `+=`, `-=`, `*=`, `/=` will be overloaded as properly. Let’s check out an instance:
Code
class Quantity:
    def __init__(self, worth):
        self.worth = worth
    def __iadd__(self, different):
        self.worth += different
        return self
num = Quantity(5)
num += 2
print(num.worth)
Output
7
Logical Operators
Logical operators like `and,` `or,` and `not` will be overloaded too. Right here’s a easy instance:
Code
class Boolean:
    def __init__(self, worth):
        self.worth = worth
    def __and__(self, different):
        return Boolean(self.worth and different.worth)
    def __or__(self, different):
        return Boolean(self.worth or different.worth)
    def __not__(self):
        return Boolean(not self.worth)
    def __repr__(self):
        return f"Boolean({self.worth})"
# Utilization
bool1 = Boolean(True)
bool2 = Boolean(False)
result_and = bool1 & bool2
print(result_and)
result_or = bool1 | bool2
print(result_or)
Output
Boolean(False)
Boolean(True)
Bitwise Operators
Bitwise operators like `&,` `|,` `^,` `<<, “>>` will also be overloaded. Let’s see an instance:
Code
class Bitwise:
    def __init__(self, worth):
        self.worth = worth
    def __and__(self, different):
        return self.worth & different.worth
bit1 = Bitwise(5)
bit2 = Bitwise(3)
consequence = bit1 & bit2
print(consequence)
Output
1
Additionally learn:Â A Full Python Tutorial to Be taught Knowledge Science from Scratch
Frequent Use Instances for Operator Overloading
Listed here are some use instances of operator overloading:
Customized Lessons
Once we create customized courses, we are able to outline particular strategies that allow us to make use of operators on objects of these courses. For instance, let’s create a category known as `Level` and overload the `+` operator so as to add two factors collectively:
Code
class Level:
    def __init__(self, x, y):
        self.x = x
        self.y = y
    def __add__(self, different):
        return Level(self.x + different.x, self.y + different.y)
p1 = Level(1, 2)
p2 = Level(3, 4)
consequence = p1 + p2
print(consequence.x, consequence.y)
Output
4 6
Mathematical Operations
We are able to additionally overload mathematical operators on customized objects like `+,` `-,` `*,` `/,` and so forth. Let’s create a category known as `Vector` and overload the `*` operator to carry out scalar multiplication:
Code
class Vector:
    def __init__(self, x, y):
        self.x = x
        self.y = y
    def __mul__(self, scalar):
        return Vector(self.x * scalar, self.y * scalar)
v = Vector(3, 4)
consequence = v * 2
print(consequence.x, consequence.y)
Output
6 8
String Concatenation
We are able to additionally overload the `+` operator to concatenate strings. Let’s create a category known as `CustomString` and overload the `+` operator to concatenate two strings:
Code
class CustomString:
    def __init__(self, worth):
        self.worth = worth
    def __add__(self, different):
        return CustomString(self.worth + different.worth)
s1 = CustomString("Howdy, ")
s2 = CustomString("World!")
consequence = s1 + s2
print(consequence.worth)
Output
Howdy, World!
Indexing and Slicing
We are able to overload the `[]` operator to allow indexing and slicing on customized objects. Let’s create a category known as `CustomList` and overload the `[]` operator to entry components by index:
Code
class CustomList:
    def __init__(self, knowledge):
        self.knowledge = knowledge
    def __getitem__(self, index):
        return self.knowledge[index]
c_list = CustomList([1, 2, 3, 4, 5])
print(c_list[2])
Output
3
Conclusion
Operator overloading emerges as a beacon of versatility in Python, orchestrating a symphony of readability, expressiveness, and domain-specificity inside codebases. Its transformative energy extends past syntax, transcending into conceptual alignment and code magnificence. Operator overloading empowers builders to sculpt options that mirror real-world situations with uncanny constancy, from arithmetic wizardry to bitwise sorcery.
As a conduit between summary notions and tangible implementations, it fosters a programming panorama the place complexity yields readability and the place customized objects seamlessly mix into the material of Pythonic constructs. Within the tapestry of Python improvement, operator overloading weaves threads of instinct, conciseness, and maintainability, elevating the craft of software program engineering to new heights of sophistication and coherence.
You may as well go for a Python course by enrolling within the – Be taught Python for Knowledge Science immediately!