Introduction
As AI is taking on the world, Massive language fashions are in big demand in know-how. Massive Language Fashions generate textual content in a method a human does. They can be utilized to develop pure language processing (NLP) purposes various from chatbots and textual content summarizers to translation apps, digital assistants, and many others.
Google launched its next-generation mannequin named Palm 2. This mannequin excels in superior scientific and mathematical operations and is utilized in reasoning and language translations. This mannequin is educated over 100+ spoken phrase languages and 20+ programming languages.
As it’s educated in varied programming languages, it may be used to translate one programming language to a different. For instance, if you wish to translate Python code to R or JavaScript code to TypeScript, and many others., you possibly can simply use Palm 2 to do it for you. Other than these, it could generate idioms and phrases and simply break up a posh activity into easier duties, making it a lot better than the earlier massive language fashions.
Studying Goals
- Introduction to Google’s Palm API
- Learn to entry Palm API by producing an API key
- Utilizing Python, learn to generate easy textual content utilizing textual content mannequin
- Learn to create a easy chatbot utilizing Python
- Lastly, we focus on how you can use Langchain with Palm API.
This text was revealed as part of the Information Science Blogathon.
Palm API
Utilizing the Palm API, you possibly can entry the capabilities of Google’s Generative AI fashions and develop fascinating AI-powered purposes. Nevertheless, if you wish to work together immediately with the Palm 2 mannequin from the browser, you need to use the browser-based IDE “MakerSuite”. However you possibly can entry the Palm 2 mannequin utilizing the Palm API to combine massive language fashions into your purposes and construct AI-driven purposes utilizing your organization’s information.
Three completely different immediate interfaces are designed, and you may get began with anyone amongst them utilizing the Palm API. They’re:
- Textual content Prompts: You should use the mannequin named “text-bison-001 to generate easy textual content. Utilizing textual content prompts, you possibly can generate textual content, generate code, edit textual content, retrieve info, extract information, and many others..
- Information Prompts: These will let you assemble prompts in a tabular format.
- Chat Prompts: Chat prompts are used to construct conversations. You should use the mannequin named “chat-bison-001” to make use of chat providers.
Entry Palm API
Navigate to the web site https://builders.generativeai.google/ and be a part of the maker suite. You’ll be added to the waitlist and can be given entry in all probability inside 24 hours.
Generate an API key:
- It’s essential to get your personal API key to make use of the API.
- You possibly can join your software to the Palm API and entry its providers utilizing the API key.
- As soon as your account is registered, it is possible for you to to generate it.
- Subsequent, go forward and generate your API key, as proven within the screenshot beneath:
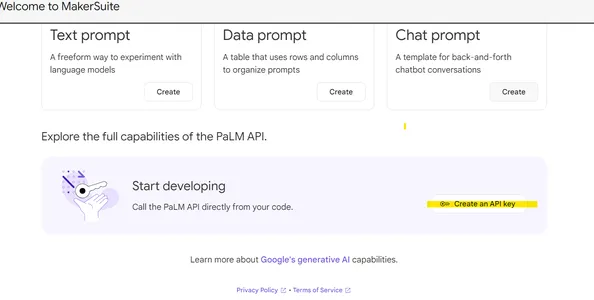
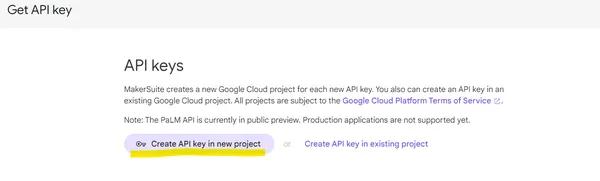
Save the API key as we’ll use it additional.
Setting the Setting
To make use of the API with Python, set up it utilizing the command:
pip set up google-generativeai
Subsequent, we configure it utilizing the API key that we generated earlier.
import google.generativeai as palm
palm.configure(api_key=API_KEY)
To checklist the out there fashions, we write the beneath code:
fashions = [model for model in palm.list_models()]
for mannequin in fashions:
print(mannequin.identify)
Output:
fashions/chat-bison-001
fashions/text-bison-001
fashions/embedding-gecko-001
Generate Textual content
We use the mannequin “text-bison-001” to generate textual content and move GenerateTextRequest. The generate_text() perform takes in two parameters i.e., a mannequin and a immediate. We move the mannequin as “text-bison-001,” and the immediate comprises the enter string.
Rationalization:
- Within the instance beneath, we move the model_id variable with the mannequin identify and a immediate variable containing the enter textual content.
- We then move the model_id as mannequin and the immediate as immediate to the generate_text() methodology.
- The temperature parameter signifies how random the response can be. In different phrases, if you’d like the mannequin to be extra inventive, you may give it a worth of 0.3.
- Lastly, the parameter “max_tokens” signifies the utmost variety of tokens the mannequin’s output can include. A token can include roughly 4 tokens. Nevertheless, for those who don’t specify, a default worth of 64 can be assigned to it.
Instance 1
model_id="fashions/text-bison-001"
immediate=""'write a canopy letter for an information science job applicaton.
Summarize it to 2 paragraphs of fifty phrases every. '''
completion=palm.generate_text(
mannequin=model_id,
immediate=immediate,
temperature=0.99,
max_output_tokens=800,
)
print(completion.end result)
Output:
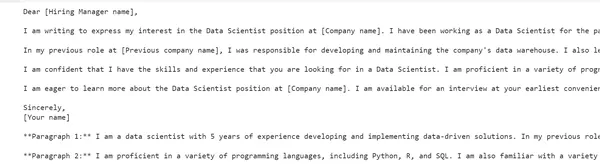
We outline some time loop that asks for enter and generates a reply. The response.final assertion prints the response.
model_id="fashions/chat-bison-001"
immediate="I need assistance with a job interview for an information analyst job. Are you able to assist me?"
examples=[
('Hello', 'Hi there mr. How can I be assistant?'),
('I want to get a High paying Job','I can work harder')
]
response=palm.chat(messages=immediate, temperature=0.2, context="Communicate like a CEO", examples=examples)
for messages in response.messages:
print(messages['author'],messages['content'])
whereas True:
s=enter()
response=response.reply(s)
print(response.final)
Output:
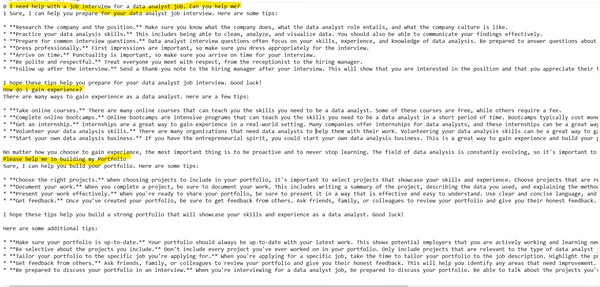
Utilizing Palm API with LangChain
LangChain is an open-source framework that lets you join massive language fashions to your purposes. To make use of Palm API with langchain, we import GooglePalmEmbeddings from langchain.embeddings. The langchain has an embedding class that gives a typical interface for varied textual content embedding fashions reminiscent of OpenAI, HuggingFace, and many others.
We move the prompts as an array, as proven within the beneath instance. Then, we name llm._generate() perform and move the prompts array as a parameter.
from langchain.embeddings import GooglePalmEmbeddings
from langchain.llms import GooglePalm
llm=GooglePalm(google_api_key=API_KEY)
llm.temperature=0.2
prompts=["How to Calculate the area of a triangle?","How many sides are there for a polygon?"]
llm_result= llm._generate(prompts)
res=llm_result.generations
print(res[0][0].textual content)
print(res[1][0].textual content)
Output:
Immediate 1
1.
**Discover the bottom and peak of the triangle.
** The bottom is the size of the facet of the triangle that's parallel to the bottom, and the peak is the size of the road phase that's perpendicular to the bottom and intersects the alternative vertex.
2.
**Multiply the bottom and peak and divide by 2.
** The method for the world of a triangle is A = 1/2 * b * h.
For instance, if a triangle has a base of 5 cm and a peak of 4 cm, its space can be 1/2 * 5 * 4 = 10 cm2.
Immediate 2
3
Conclusion
On this article, we have now launched Google’s newest Palm 2 mannequin and the way it’s higher than the earlier fashions. We then discovered how you can use Palm API with Python Programming Language. We then mentioned how you can develop easy purposes and generate textual content and chats. Lastly, we coated how you can embed it utilizing Langchain framework.
Key Takeaways
- Palm API permits customers to develop purposes utilizing Massive Language Fashions
- Palm API supplies a number of text-generation providers, reminiscent of textual content service to generate textual content and a chat service to generate chat conversations.
- Google generative-ai is the Palm API Python Library and will be simply put in utilizing the pip command.
Regularly Requested Questions
A. To shortly get began with palm API in python, you possibly can set up a library utilizing the pip command – pip set up google generative-ai.
A. Sure, you possibly can entry Google’s Massive Language Fashions and develop purposes utilizing Palm API.
A. Sure, Google’s Palm API and MakerSuite can be found for public preview.
A. Google’s Palm 2 mannequin was educated in over 20 programming languages and may generate code in varied programming languages.
A. Palm API comes with each textual content and chat providers. It supplies a number of textual content technology capabilities.
The media proven on this article just isn’t owned by Analytics Vidhya and is used on the Creator’s discretion.