Introduction
Earlier than the massive language fashions period, extracting invoices was a tedious job. For bill extraction, one has to collect knowledge, construct a doc search machine studying mannequin, mannequin fine-tuning and many others. The introduction of Generative AI took all of us by storm and plenty of issues had been simplified utilizing the LLM mannequin. The big language mannequin has eliminated the model-building technique of machine studying; you simply must be good at immediate engineering, and your work is finished in a lot of the situation. On this article, we’re making an bill extraction bot with the assistance of a giant language mannequin and LangChain. Nevertheless, the detailed information of LangChain and LLM is out of scope however beneath is a brief description of LangChain and its parts.
Studying Goals
- Learn to extract info from a doc
- Tips on how to construction your backend code through the use of LangChain and LLM
- Tips on how to present the precise prompts and directions to the LLM mannequin
- Good information of Streamlit framework for front-end work
This text was revealed as part of the Knowledge Science Blogathon.
What’s a Giant Language Mannequin?
Giant language fashions (LLMs) are a sort of synthetic intelligence (AI) algorithm that makes use of deep studying methods to course of and perceive pure language. LLMs are skilled on huge volumes of textual content knowledge to find linguistic patterns and entity relationships. Because of this, they’ll now acknowledge, translate, forecast, or create textual content or different info. LLMs could be skilled on doable petabytes of knowledge and could be tens of terabytes in dimension. As an illustration, one gigabit of textual content house might maintain round 178 million phrases.
For companies wishing to supply buyer help by way of a chatbot or digital assistant, LLMs could be useful. With out a human current, they’ll provide individualized responses.
What’s LangChain?
LangChain is an open-source framework used for creating and constructing functions utilizing a big language mannequin (LLM). It offers an ordinary interface for chains, many integrations with different instruments, and end-to-end chains for frequent functions. This allows you to develop interactive, data-responsive apps that use the latest advances in pure language processing.
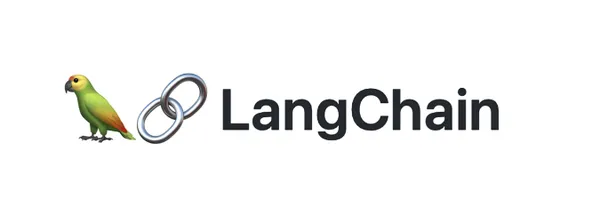
Core Elements of LangChain
Quite a lot of Langchain’s parts could be “chained” collectively to construct advanced LLM-based functions. These parts include:
- Immediate Templates
- LLMs
- Brokers
- Reminiscence
Constructing Bill Extraction Bot utilizing LangChain and LLM
Earlier than the period of Generative AI extracting any knowledge from a doc was a time-consuming course of. One has to construct an ML mannequin or use the cloud service API from Google, Microsoft and AWS. However LLM makes it very straightforward to extract any info from a given doc. LLM does it in three easy steps:
- Name the LLM mannequin API
- Correct immediate must be given
- Info must be extracted from a doc
For this demo, we now have taken three bill pdf information. Beneath is the screenshot of 1 bill file.
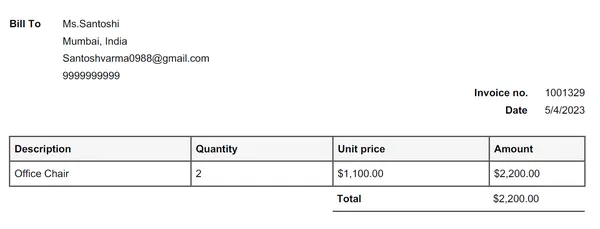
Step 1: Create an OpenAI API Key
First, you need to create an OpenAI API key (paid subscription). One can discover simply on the web, how one can create an OpenAI API key. Assuming the API secret’s created. The following step is to put in all the required packages reminiscent of LangChain, OpenAI, pypdf, and many others.
#putting in packages
pip set up langchain
pip set up openai
pip set up streamlit
pip set up PyPDF2
pip set up pandas
Step 2: Importing Libraries
As soon as all of the packages are put in. It’s time to import them one after the other. We’ll create two Python information. One accommodates all of the backend logic (named “utils.py”), and the second is for creating the entrance finish with the assistance of the streamlit bundle.
First, we’ll begin with “utils.py” the place we’ll create a number of features.
#import libraries
from langchain.llms import OpenAI
from pypdf import PdfReader
import pandas as pd
import re
from langchain.llms.openai import OpenAI
from langchain.prompts import PromptTemplate
Let’s create a operate which extracts all the data from a PDF file. For this, we’ll use the PdfReader bundle:
#Extract Info from PDF file
def get_pdf_text(pdf_doc):
textual content = ""
pdf_reader = PdfReader(pdf_doc)
for web page in pdf_reader.pages:
textual content += web page.extract_text()
return textual content
Then, we’ll create a operate to extract all of the required info from an bill PDF file. On this case, we’re extracting Bill No., Description, Amount, Date, Unit Value, Quantity, Complete, Electronic mail, Cellphone Quantity, and Deal with and calling OpenAI LLM API from LangChain.
def extract_data(pages_data):
template=""'Extract all following values: bill no., Description,
Amount, date, Unit value, Quantity, Complete,
e mail, cellphone quantity and tackle from this knowledge: {pages}
Anticipated output : take away any greenback symbols {{'Bill no.':'1001329',
'Description':'Workplace Chair', 'Amount':'2', 'Date':'05/01/2022',
'Unit value':'1100.00', Quantity':'2200.00', 'Complete':'2200.00',
'e mail':'[email protected]', 'cellphone quantity':'9999999999',
'Deal with':'Mumbai, India'}}
'''
prompt_template = PromptTemplate(input_variables=['pages'], template=template)
llm = OpenAI(temperature=0.4)
full_response = llm(prompt_template.format(pages=pages_data))
return full_response
Step 5: Create a Perform that may Iterate by all of the PDF Information
Writing one final operate for the utils.py file. This operate will iterate by all of the PDF information which suggests you possibly can add a number of bill information at one go.
# iterate over information in
# that person uploaded PDF information, one after the other
def create_docs(user_pdf_list):
df = pd.DataFrame({'Bill no.': pd.Collection(dtype="str"),
'Description': pd.Collection(dtype="str"),
'Amount': pd.Collection(dtype="str"),
'Date': pd.Collection(dtype="str"),
'Unit value': pd.Collection(dtype="str"),
'Quantity': pd.Collection(dtype="int"),
'Complete': pd.Collection(dtype="str"),
'Electronic mail': pd.Collection(dtype="str"),
'Cellphone quantity': pd.Collection(dtype="str"),
'Deal with': pd.Collection(dtype="str")
})
for filename in user_pdf_list:
print(filename)
raw_data=get_pdf_text(filename)
#print(raw_data)
#print("extracted uncooked knowledge")
llm_extracted_data=extracted_data(raw_data)
#print("llm extracted knowledge")
#Including objects to our checklist - Including knowledge & its metadata
sample = r'{(.+)}'
match = re.search(sample, llm_extracted_data, re.DOTALL)
if match:
extracted_text = match.group(1)
# Changing the extracted textual content to a dictionary
data_dict = eval('{' + extracted_text + '}')
print(data_dict)
else:
print("No match discovered.")
df=df.append([data_dict], ignore_index=True)
print("********************DONE***************")
#df=df.append(save_to_dataframe(llm_extracted_data), ignore_index=True)
df.head()
return df
Until right here our utils.py file is accomplished, Now it’s time to begin with the app.py file. The app.py file accommodates front-end code with the assistance of the streamlit bundle.
Streamlit Framework
An open-source Python app framework known as Streamlit makes it simpler to construct net functions for knowledge science and machine studying. You may assemble apps utilizing this technique in the identical manner as you write Python code as a result of it was created for machine studying engineers. Main Python libraries together with scikit-learn, Keras, PyTorch, SymPy(latex), NumPy, pandas, and Matplotlib are appropriate with Streamlit. Working pip will get you began with Streamlit in lower than a minute.
Set up and Import all Packages
First, we’ll set up and import all the required packages
#importing packages
import streamlit as st
import os
from dotenv import load_dotenv
from utils import *
Create the Essential Perform
Then we’ll create a primary operate the place we’ll point out all of the titles, subheaders and front-end UI with the assistance of streamlit. Consider me, with streamlit, it is extremely easy and straightforward.
def primary():
load_dotenv()
st.set_page_config(page_title="Bill Extraction Bot")
st.title("Bill Extraction Bot...💁 ")
st.subheader("I will help you in extracting bill knowledge")
# Add the Invoices (pdf information)
pdf = st.file_uploader("Add invoices right here, solely PDF information allowed",
kind=["pdf"],accept_multiple_files=True)
submit=st.button("Extract Knowledge")
if submit:
with st.spinner('Look forward to it...'):
df=create_docs(pdf)
st.write(df.head())
data_as_csv= df.to_csv(index=False).encode("utf-8")
st.download_button(
"Obtain knowledge as CSV",
data_as_csv,
"benchmark-tools.csv",
"textual content/csv",
key="download-tools-csv",
)
st.success("Hope I used to be in a position to save your time❤️")
#Invoking primary operate
if __name__ == '__main__':
primary()
Run streamlit run app.py
As soon as that’s carried out, save the information and run the “streamlit run app.py” command within the terminal. Bear in mind by default streamlit makes use of port 8501. You can even obtain the extracted info in an Excel file. The obtain choice is given within the UI.
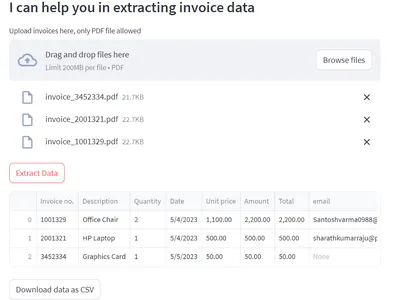
Conclusion
Congratulations! You could have constructed a tremendous and time-saving app utilizing a big language mannequin and streamlit. On this article, we now have discovered what a big language mannequin is and the way it’s helpful. As well as, we now have discovered the fundamentals of LangChain and its core parts and a few functionalities of the streamlit framework. An important a part of this weblog is the “extract_data” operate (from the code session), which explains how one can give correct prompts and directions to the LLM mannequin.
You could have additionally discovered the next:
- Tips on how to extract info from an bill PDF file.
- Use of streamlit framework for UI
- Use of OpenAI LLM mannequin
This offers you some concepts on utilizing the LLM mannequin with correct prompts and directions to satisfy your job.
Continuously Requested Query
A. Streamlit is a library which lets you construct the entrance finish (UI) to your knowledge science and machine studying duties by writing all of the code in Python. Lovely UIs can simply be designed by quite a few parts from the library.
A. Flask is a light-weight micro-framework that’s easy to study and use. A newer framework known as Streamlit is made completely for net functions which might be pushed by knowledge.
A. No, It is determined by the use case to make use of case. On this instance, we all know what info must be extracted however if you wish to extract roughly info you have to give the correct directions and an instance to the LLM mannequin accordingly it would extract all of the talked about info.
A. Generative AI has the potential to have a profound influence on the creation, development, and play of video video games in addition to it may substitute most human-level duties with automation.
The media proven on this article just isn’t owned by Analytics Vidhya and is used on the Creator’s discretion.