As a developer, you’re seemingly subscribed to a dozen newsletters, blogs, and RSS feeds, all competing on your consideration. Once you’re centered on constructing tasks or studying new tech, discovering time to sift by all that content material is usually a problem.
What if AI brokers curated all of your newsletters into one?
On this tutorial, you’ll learn to construct an AI-powered publication aggregator utilizing React and KaibanJS. By establishing a number of AI brokers, you’ll automate the method of:
- Fetching articles from varied newsletters.
- Filtering content material primarily based in your pursuits and matters
- Aggregating all the pieces right into a single, easy-to-read format all inside your JavaScript atmosphere.
With KaibanJS, you’ll benefit from AI Brokers that deal with every step of the method. Better of all, you’ll be capable of set this up in simply 10 minutes, making it a fast and highly effective addition to your improvement toolkit.
KaibanJS is a brand new open-source JavaScript framework for constructing multi-agent programs.
By the top of this tutorial, you’ll have a completely practical publication aggregator that retains you up to date on the matters that matter to you, routinely.
Give it a strive first: Discover this dwell demonstration of the AI brokers in motion! This instance will present a hands-on take a look at how the brokers work collectively to collect, filter, and current publication content material. Dive in to get a really feel for the method earlier than you begin constructing your individual setup on this 10-minute tutorial. 😅
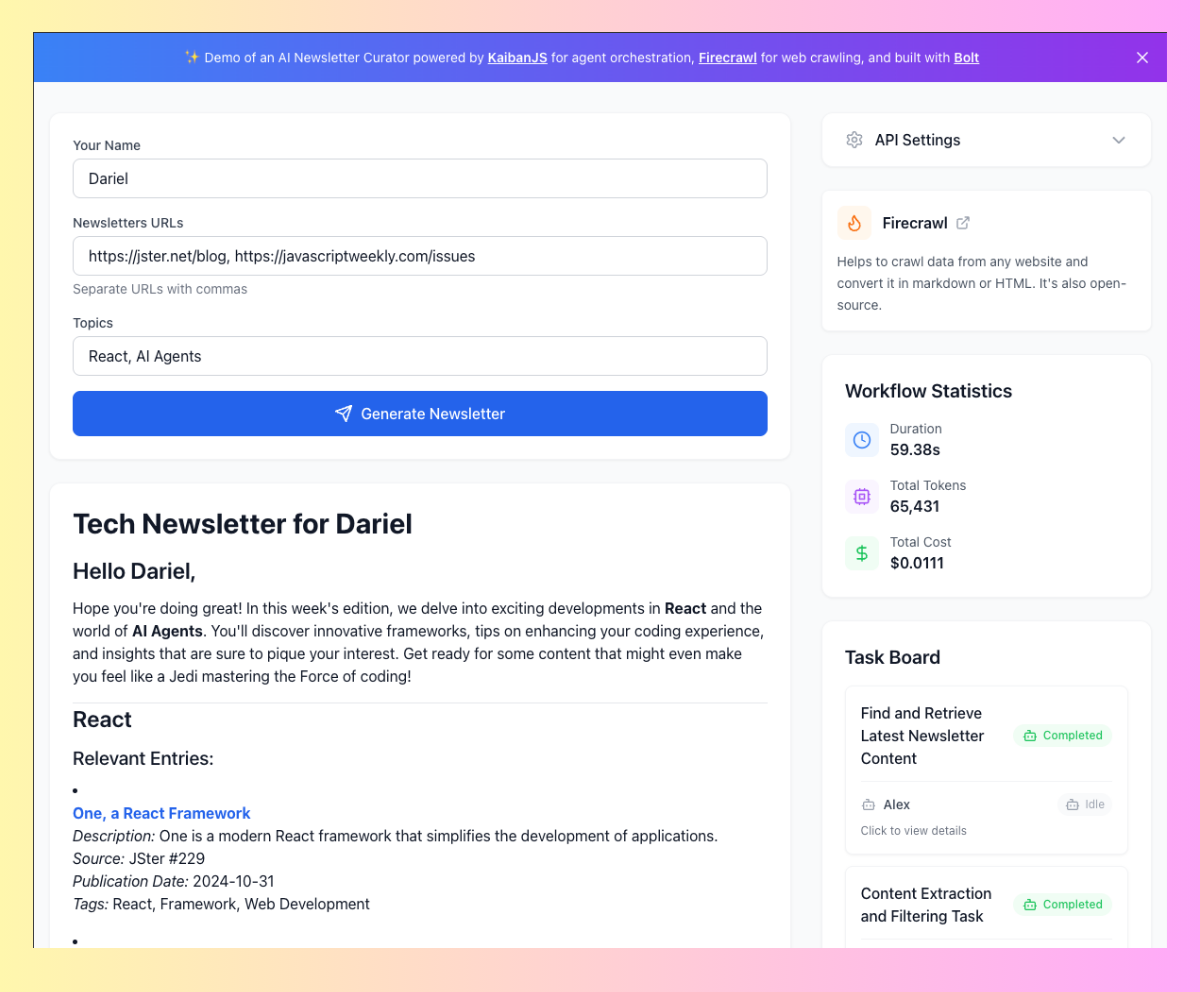
Fast Navigation
Understanding AI Brokers
In the event you’re not accustomed to AI brokers or AI on the whole, don’t fear! They could sound advanced, however they’re basically sensible instruments that may self-automate repetitive duties for you.
Let’s break it down:
1) What’s an AI Agent?
An AI agent is sort of a self-sufficient operate that may deal with duties by itself. When you give it a job or a objective, the agent works autonomously to get it accomplished. For instance, an agent might:
- Plan the best way to accumulate the information.
- Determine if it must run internet searches or pull from an API.
- Write a draft primarily based on the information it discovered.
- Evaluate the draft for enhancements.
- Revise the content material to make it higher.
The hot button is that the agent does all of this independently, with out you having to handle every step. It’s like having a really specialised co-worker in a given activity that won’t cease till the duty is completed.
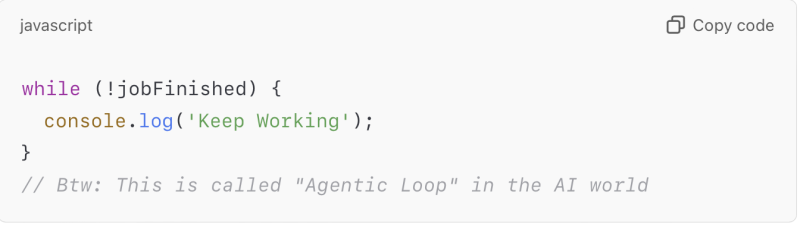
2) How do Brokers work?
Right here’s a fast video that provides you an outline of the principle ideas, together with how KaibanJS brokers execute duties, use instruments, and collaborate to deal with workflows.
1. Brokers Execute Duties
Every agent is assigned a particular activity to finish. These duties might be so simple as gathering information from an online API or as advanced as producing summaries or making selections. As soon as a activity is given, the agent works autonomously to complete it with out additional directions.
2. Brokers Use Instruments
Brokers typically want entry to exterior instruments to finish their duties. These instruments might be APIs, databases, and even internet searches. For instance, a Researcher Agent may use a search API to collect articles, whereas a Author Agent might use a language mannequin to summarize the content material.
3. Brokers Collaborate with Different Brokers
In KaibanJS, brokers don’t work alone—they will collaborate with different brokers. For instance, a Researcher Agent may collect information, and as soon as the information is prepared, a Author Agent can step in to create a abstract. This lets you break advanced workflows into smaller, manageable duties, every dealt with by specialised brokers.
3) What Can You Construct with A number of Brokers?
Listed below are some examples Javascript builders can construct utilizing a number of brokers:
- An AI code editor like Cursor AI, which assists you in writing and debugging code with AI.
- An AI-powered search assistant like Perplexity AI, the place a crew of brokers retrieves, processes, and offers concise solutions from a number of sources.
- A CI/CD pipeline agent that critiques pull requests, checks code high quality, and suggests enhancements to take care of excessive requirements in your tasks.
Now that you simply perceive how AI brokers work and what makes them so highly effective, it’s time to get hands-on. Let’s arrange KaibanJS and begin constructing your first agent-driven workflow.
Setup
To get began, clone the demo repository from GitHub:
git clone https://github.com/kaiban-ai/kaiban-agents-aggregator
Then, navigate to your challenge listing and run the next command in your terminal:
npx kaibanjs@newest init
This command will set up KaibanJS in your challenge and arrange a primary construction. It should additionally open the Kaiban Board in your browser, the place you’ll be able to visually handle brokers and duties (sort of a cute playground).
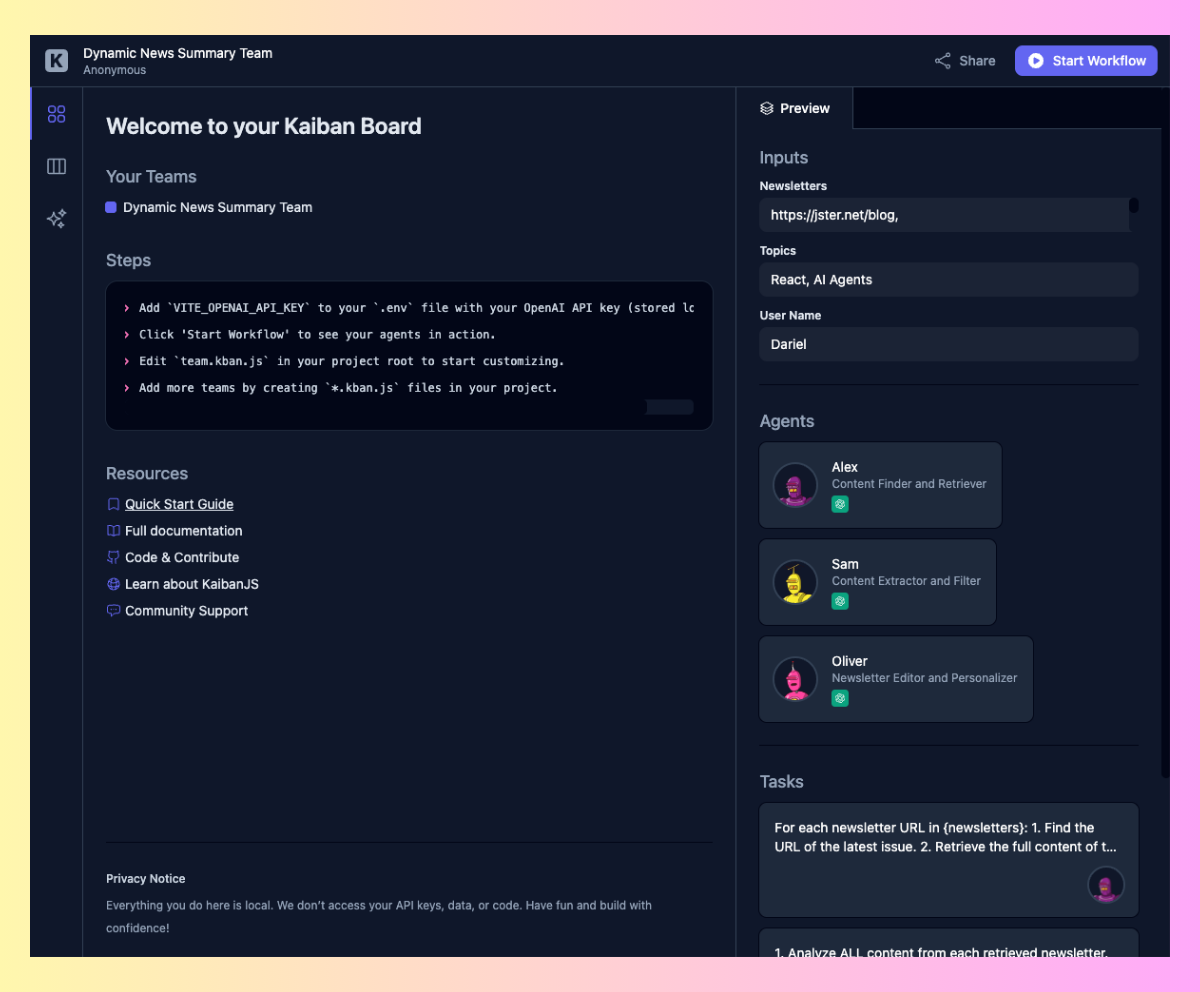
To allow particular brokers (just like the content material retrieval agent) to make use of instruments that require API keys, create a .env
file in your challenge’s root listing and add your keys there:
VITE_FIRECRAWL_API_KEY=your_firecrawl_api_key
VITE_OPENAI_API_KEY=your_openai_api_key
The place to get the API keys:
- Firecrawl API Key: Join at Firecrawl to create an account and procure an API key for internet scraping capabilities.
- OpenAI API Key: Go to OpenAI’s web site to create an account and procure an API key, which can enable brokers to make use of language processing capabilities with OpenAI fashions.
Create your crew of Brokers
Open crew.kban.js in your challenge root. Let’s stroll by every a part of the system to know the way it works.
1. Preliminary Setup and Instruments
First, we import our dependencies and arrange the online scraping instrument:
import { Agent, Job, Workforce } from 'kaibanjs';
import { Firecrawl } from '@kaibanjs/instruments';
// Initialize the Firecrawl instrument
const firecrawlTool = new Firecrawl({
apiKey: import.meta.env.VITE_FIRECRAWL_API_KEY
});
The Firecrawl instrument (firecrawl.dev) is important for fetching content material from varied web sites in a digestible format for LLMs. It requires an API key which you’ll acquire on their web site. (You may construct this your self utilizing their open supply model or puppeteer… however I used to be simply too lazy 😅)
2. Defining Our Brokers
We create three specialised brokers, every with distinctive capabilities:
- Alex fetches uncooked content material utilizing the Firecrawl instrument (Discover how Alex is sensible sufficient to routinely get the newest publication concern from the problems record…)
- Sam analyzes and filters the content material primarily based on consumer pursuits
- Oliver transforms the filtered content material into a refined publication
// Outline Brokers
const contentRetrievalAgent = new Agent({
identify: 'Alex',
function: 'Content material Finder and Retriever',
objective: 'Discover the newest problems with offered newsletters and retrieve their content material.',
background: 'Internet Scraping, URL Extraction, and Content material Retrieval',
instruments: [firecrawlTool],
maxIterations: 15,
});
const contentExtractorAgent = new Agent({
identify: 'Sam',
function: 'Content material Extractor and Filter',
objective: `Extract and filter info associated to specified matters from
the retrieved publication content material.`,
background: 'Content material Evaluation, Info Extraction, and Subject Filtering',
instruments: [],
});
const editorAgent = new Agent({
identify: 'Oliver',
function: 'Publication Editor and Personalizer',
objective: `Format the extracted content material right into a publication with a personalised,
participating introduction.`,
background: 'Content material Group, Publication Writing, and Personalization',
instruments: [],
});
3. Creating Particular Duties
Every agent wants clear directions about what to perform:
// Outline Duties
const contentRetrievalTask = new Job({
title: 'Discover and Retrieve Newest Publication Content material',
description: `
For every publication URL in {newsletters}:
1. Discover the URL of the newest concern.
2. Retrieve the total content material of the newest concern.
Return the content material from all newsletters.
`,
expectedOutput: 'Uncooked content material from the newest problems with all offered newsletters.',
agent: contentRetrievalAgent,
});
const contentExtractionTask = new Job({
title: 'Content material Extraction and Filtering Job',
description: `
1. Analyze ALL content material from every retrieved publication.
2. Extract ANY and ALL info associated to the offered matters: {matters}.
3. Use a broad interpretation of relevance to make sure no doubtlessly related objects are missed.
4. For every extracted merchandise, seize:
- Full article title with its URL
- Full unique description or snippet
- Publication supply identify
- Publication date
- Any related tags, classes, or key phrases
5. Group the extracted info by subject. An merchandise can seem underneath a number of matters if related.
6. If an merchandise's relevance is unsure, embrace it and be aware the uncertainty.
`,
expectedOutput: 'Structured information containing all extracted and filtered content material, grouped by subject.',
agent: contentExtractorAgent,
});
const editingTask = new Job({
title: 'Publication Modifying and Personalization Job',
description: `
1. Format the extracted content material right into a publication construction.
2. Create a personalised, participating introduction for {userName}:
- Begin with a pleasant greeting utilizing the consumer's identify.
- Briefly point out the matters lined ({matters}).
- Tease some fascinating content material or traits from the articles.
- Optionally, embrace a delicate nerdy reference (ideally Star Wars-related) that matches naturally.
- Hold the tone skilled but pleasant, appropriate for a tech publication.
3. Set up the content material by matters, with every subject as a bit.
4. Underneath every subject, record the related entries with all captured info.
5. Add a abstract of merchandise counts for every subject and publication on the finish.
`,
expectedOutput: 'An entire, markdown-formatted publication',
agent: editorAgent,
});
Discover how every activity:
- Has a transparent title and detailed directions
- Makes use of placeholders like
{newsletters}
,{matters}
, and{userName}
which can be stuffed with consumer inputs - Specifies what output to count on
- Is assigned to the suitable agent
4. Constructing the Workforce
Lastly, we assemble all the pieces right into a coordinated crew:
// Outline the Workforce
export const newsSummaryTeam = new Workforce({
identify: 'Dynamic Information Abstract Workforce',
brokers: [contentRetrievalAgent, contentExtractorAgent, editorAgent],
duties: [contentRetrievalTask, contentExtractionTask, editingTask],
inputs: {
newsletters: '', // Can be stuffed with publication URLs
matters: '', // Can be stuffed with consumer's pursuits
userName: '', // Can be stuffed with consumer's identify
},
env: {
OPENAI_API_KEY: import.meta.env.VITE_OPENAI_API_KEY,
},
});
The crew configuration:
- Lists all brokers and their duties within the order they’ll execute
- Supplies placeholders for consumer inputs
- Units up essential atmosphere variables
💡 You will discover the total implementation in our GitHub repository, together with extra options and optimizations!
You may run the crew programmatically and deal with its output by the console or any API:
// Begin the workflow programmatically
crew.begin({
newsletters: 'https://newsletter1.com,https://newsletter2.com',
matters: 'React, AI',
userName: 'Dev'
})
.then((output) => {
console.log("Workflow accomplished:", output.outcome);
})
.catch((error) => {
console.error("Workflow error:", error);
});
For this tutorial although, let’s visualize our workflow utilizing the Kaiban Board.
Your Workforce within the Kaiban Board
Now that you simply’ve configured your crew, you’ll see your publication aggregation workflow come to life.
Bear in mind the Kaiban Board that opened if you ran npx kaibanjs@newest init
? In the event you closed your terminal or have to restart the board, merely run:
npm run kaiban
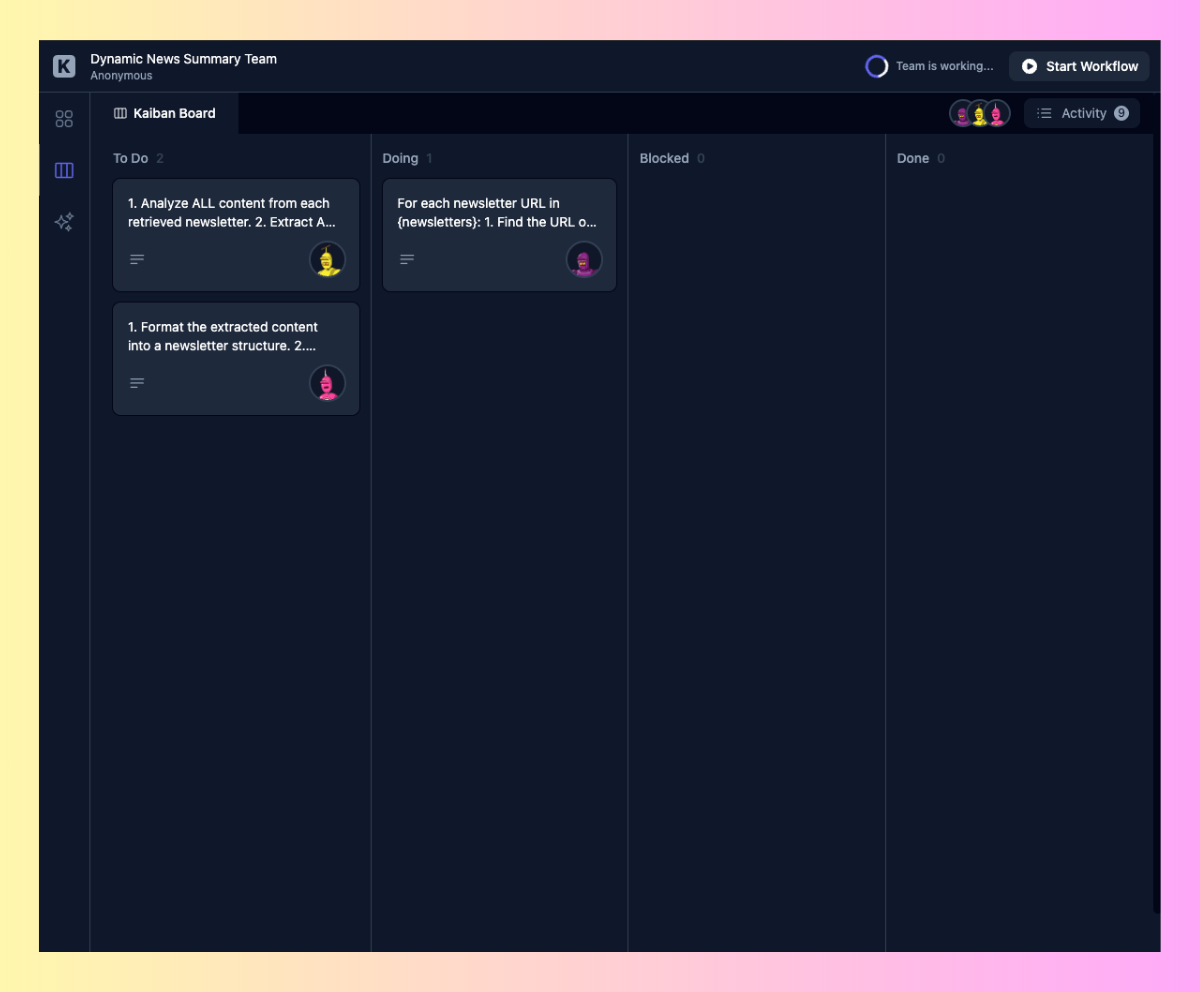
The board shows your three duties properly organized within the “To Do” column:
- Alex’s 👤 content material retrieval activity – able to fetch publication content material
- Sam’s 👤 extraction activity – ready to filter and arrange info
- Oliver’s 👤 modifying activity – ready to create your customized publication
As you click on “Begin Workflow”, you’ll see these duties transfer by the columns from
“To Do” → “Doing” → “Achieved”, together with your brokers working collectively to create your publication.
💡 Watch the board replace in real-time as your brokers work. You may click on on any activity to see particulars about what every agent is doing!
Now that your crew is ready up and visual within the Kaiban Board, let’s transfer on to…
Constructing a Customized UI with React
Whereas the Kaiban Board is nice for experimenting and collaborating together with your crew, likelihood is you’ll wish to create a customized UI utilizing React or another frontend framework. Let’s construct one collectively!
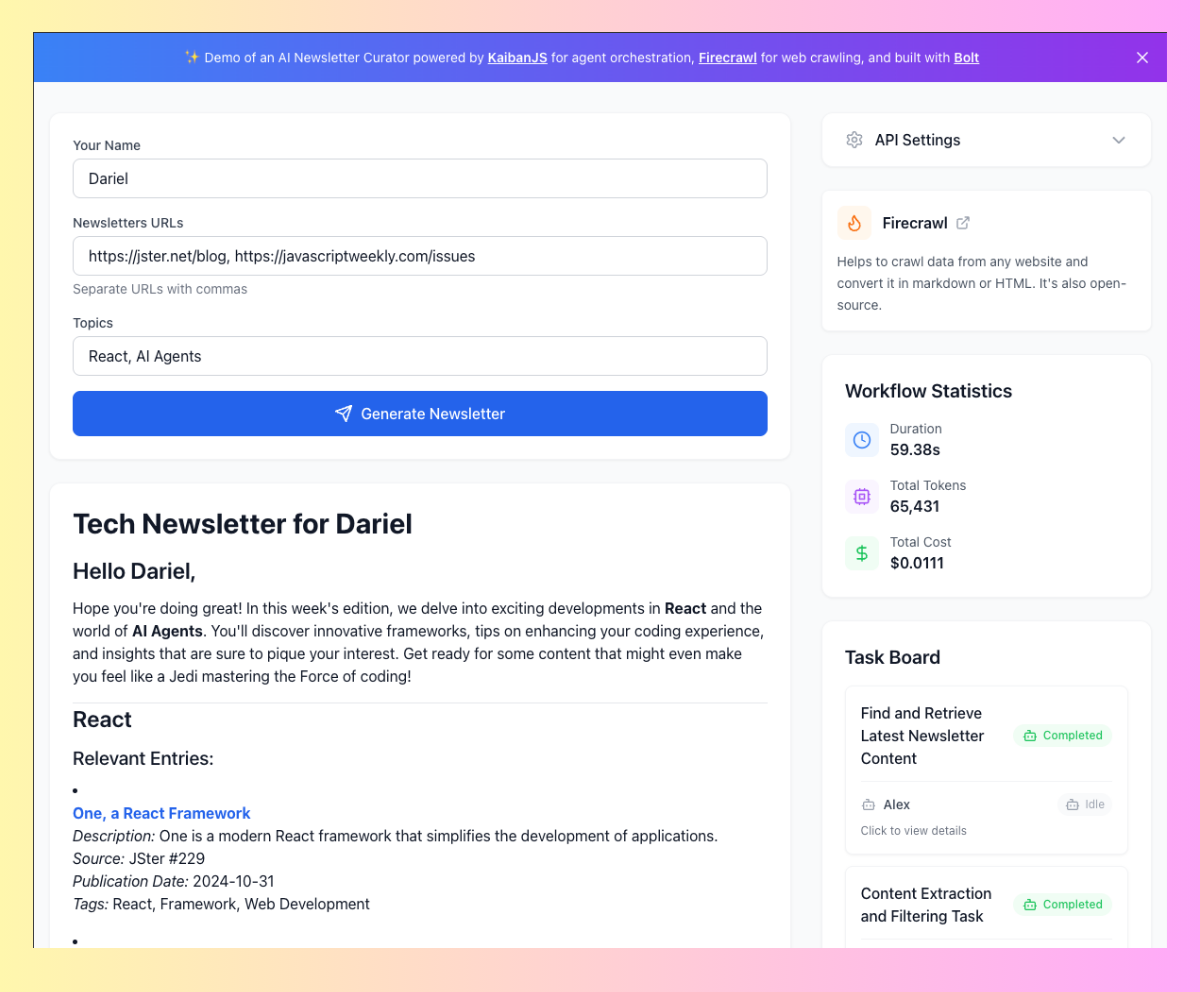
Need to see the entire implementation?
Step 1: Primary Setup
First, create a brand new React element and join it to our KaibanJS retailer:
import React, { useState } from 'react';
import newsSummaryTeam from '../crew.kban.js';
import ReactMarkdown from 'react-markdown';
operate NewsletterApp() {
const useTeamStore = newsSummaryTeam.useStore();
const { teamWorkflowStatus, workflowResult, inputs } = useTeamStore(state => ({
teamWorkflowStatus: state.teamWorkflowStatus,
workflowResult: state.workflowResult,
inputs: state.inputs
}));
const [topic, setTopic] = useState(inputs.matters);
const [newsletters, setNewsletters] = useState(inputs.newsletters);
return (
<div>
{/* We'll add our UI right here */}
</div>
);
}
Step 2: Including Consumer Inputs
Let’s add inputs for matters and publication URLs:
operate NewsletterApp() {
const useTeamStore = newsSummaryTeam.useStore();
const { teamWorkflowStatus, workflowResult, inputs } = useTeamStore(state => ({
teamWorkflowStatus: state.teamWorkflowStatus,
workflowResult: state.workflowResult,
inputs: state.inputs
}));
const [topic, setTopic] = useState(inputs.matters);
const [newsletters, setNewsletters] = useState(inputs.newsletters);
const [userName, setUserName] = useState(inputs.userName);
const [workflowStats, setWorkflowStats] = useState(null);
const generateNewsletter = async () => {
strive {
setWorkflowStats(null);
const output = await newsSummaryTeam.begin( 'Visitor'
);
// Different code...
} catch (error) {
console.error('Error producing publication:', error);
}
};
return (
<kind>
<label htmlFor="newsletters">Newsletters URLs</label>
<enter
id="newsletters"
kind="textual content"
worth={newsletters}
onChange={(e) => setNewsletters(e.goal.worth)}
placeholder="Enter publication URLs (comma-separated)..."
/>
<label htmlFor="matters">Subjects</label>
<enter
id="matters"
kind="textual content"
worth={subject}
onChange={(e) => setTopic(e.goal.worth)}
placeholder="E.g. 'ReactJS, NextJS'"
/>
<button
onClick={generateNewsletter}
disabled= !subject
>
<Ship />
Generate Publication
</button>
</kind>
);
}
Step 3: Monitoring Brokers and Duties
Add real-time monitoring of your brokers and duties:
operate NewsletterApp() {
// ... earlier code ...
// Add these to your retailer connection
const { brokers, duties } = useTeamStore(state => ({
brokers: state.brokers,
duties: state.duties
}));
return (
<div className="max-w-4xl mx-auto p-6">
{/* Earlier inputs */}
<div className="mt-6 grid grid-cols-2 gap-4">
{/* Brokers Standing */}
<div>
<h3 className="font-medium mb-2">Brokers</h3>
{brokers.map(agent => (
<div key={agent.id} className="p-2 bg-gray-50 rounded mb-2">
{agent.identify}: {agent.standing}
</div>
))}
</div>
{/* Duties Standing */}
<div>
<h3 className="font-medium mb-2">Duties</h3>
{duties.map(activity => (
<div key={activity.id} className="p-2 bg-gray-50 rounded mb-2">
{activity.title}: {activity.standing}
</div>
))}
</div>
</div>
</div>
);
}
Step 4: Displaying the Publication
Lastly, let’s add the publication show:
operate NewsletterApp() {
// ... earlier code ...
return (
<div className="max-w-4xl mx-auto p-6">
{/* Earlier sections */}
<div className="mt-6 prose max-w-none">
{workflowResult ? (
<ReactMarkdown>{workflowResult}</ReactMarkdown>
) : (
<p className="text-gray-500 text-center">
No publication generated but. Enter matters and click on 'Generate'.
</p>
)}
</div>
</div>
);
}
export default NewsletterApp;
Need to Add Extra Options?
This instance exhibits the essential implementation, however there’s way more you’ll be able to add! The entire model in our GitHub repository contains extra options like:
- Workflow statistics monitoring
- Detailed logs show
- API key administration
- Superior error dealing with
- Enhanced UI with Tailwind CSS
💡 To dive deeper browse the repository to see how every function is applied.
Wrapping Up
We’ve explored the best way to construct an AI-powered publication aggregator that transforms the best way we devour publication content material. By combining KaibanJS’s agent orchestration capabilities with React, we’ve created a instrument that routinely curates and presents content material that issues to us.
The most effective half? That is only the start of what you’ll be able to construct with AI brokers. Contemplate extending this challenge by:
- Including assist for various content material sources
- Creating customized publication templates
- Implementing computerized scheduling
- Constructing customized advice programs
We’d love to listen to about your expertise! Be at liberty to share your setup, any challenges you confronted, or customizations you added. Go away a remark beneath or tag us on social media to showcase your challenge!
KaibanJS is an open-source challenge, and we’d love your assist in making it even higher! Star the repository, report bugs, submit pull requests, or assist us to enhance the documentation.
Each contribution, irrespective of how small, helps make AI improvement extra accessible to everybody.
Imagine in love ✌️