Within the coming years there’ll undoubtedly be a shift in how all code is written. Understanding how giant studying fashions (LLM’s) work, and the way to finest work together with them by a immediate will probably be a essential talent to have. Many builders and non-developers are doing this now as a result of it’s extremely helpful to have the flexibility to generate code on demand.
I’ve been experimenting with code technology for the previous 2 years, and over the previous 6 months, it’s taken a big leap ahead. I count on this may grow to be a typical sample as time goes on, however at the same time as fashions advance there’ll at all times be elementary core ideas for interacting with an AI immediate like ChatGPT or Claude.
This has grow to be referred to as immediate engineering, and I’ll share a number of the approaches and methods I’ve discovered significantly helpful whereas producing PHP, SASS, JS, and HTML code for WordPress websites. The identical ideas can simply apply to another CMS, or growth framework as nicely.
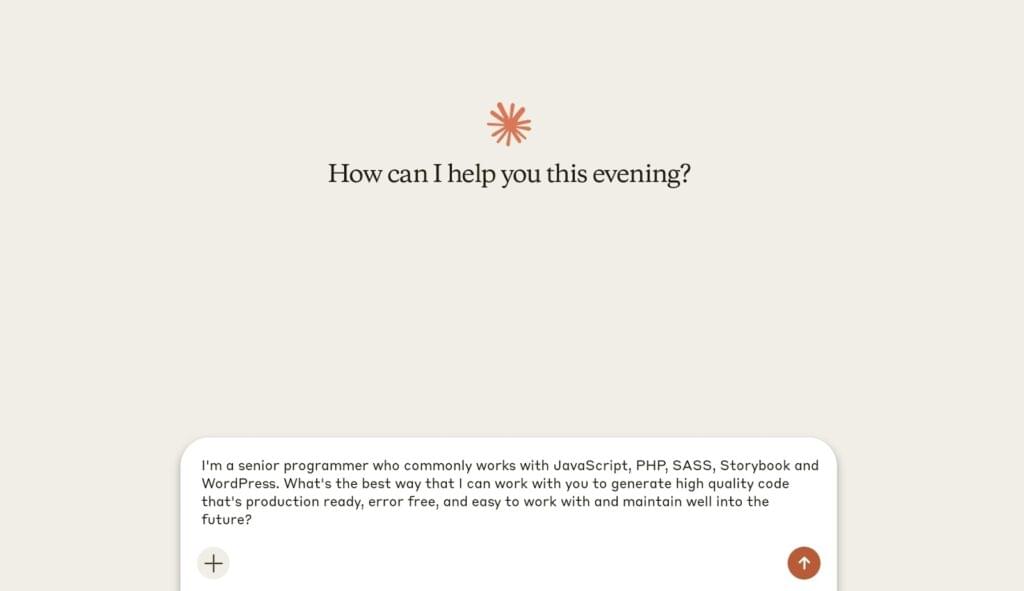
You Get What You Give
A very powerful rule to recollect when interacting with an AI immediate for code technology is that the standard of the enter you present is immediately associated to the standard of the output. All the time bear in mind you’re not describing a process to an individual, which can sound apparent however it’s a brand new idea that we don’t even understand we do.
While you ask an AI like ChatGPT or Anthropic’s Claude to put in writing code, you need to be tremendous clear. A human can guess what you imply, ask questions, and repair errors on their very own. An AI gained’t do this.
It solely follows the phrases you sort. If you happen to go away one thing out, the AI gained’t understand it’s lacking. You may know and infer issues that assume a fundamental understanding of sure features of WordPress. Issues that you just’d by no means embrace when describing a process to a different individual.
For a Individual
Are you able to modify submit titles in order that weblog posts have 'Prefix: ' earlier than them, however pages keep the identical? Ensure it is correctly escaped to keep away from safety points
For an AI Immediate
write a WordPress perform that modifies all submit titles utilizing built-in features utilizing the_title filter so as to add 'Prefix: ' earlier than the title. It will solely be utilized to posts (not pages)
It is a very rudimentary instance, and under no circumstances would I think about the AI immediate above to be a superb one. However when in comparison with the request to an individual some key features will lead to a much better reply:
- Mentioning WordPress offers the immediate with an essential contextual element
- Utilizing built-in features tells the mannequin the place to focus first to search out options
- Offering
the_title filter
hook tells the mannequin exactly the place and the way to execute this code in one of the simplest ways doable
Collectively these three features drastically slender down the main target of the mannequin, permitting it to focus much more consideration on the core process at hand. The result’s the next high quality output as compared.
Code Group & Reuse
Step one in the direction of writing extremely efficient AI prompts for producing code is to have a agency grasp on the programs, instruments, APIs, and approaches which might be finest for attaining the perfect finish outcome.
Nicely-crafted prompts that generate code, whether or not for WordPress or another programming framework or language, make good use of the present codebase within the easiest methods doable.
AI-generated code can shortly get out of hand if every perform or block of code operates impartial of all others. You’ll end up with a codebase that’s riddled with redundancy, making it bloated, much less testable, and usually rather more troublesome to work with.
Within the coming years I predict that clear, organized code will probably be a serious benefit that’s hardly ever seen. By nature, AI is not going to re-use features of your current codebase if it isn’t conscious of them.
Finally, the enter context dimension will grow to be giant sufficient to just accept a whole codebase as enter, and that may assist. However even then your codebase will must be nicely organized and comprehensible. Having a D.R.Y (Don’t repeat your self) mindset is and at all times will probably be on the crux of strong programming, whether or not it’s crafted by people or AI.
It’s totally doable to generate code with little to no topic space data, however the outcomes are more likely to trigger issues sooner or later. An AI-fueled codebase that’s been constructed piecemeal will probably be extra liable to bugs, and more durable to know. Most essential although, these points will proceed to compound as time goes on.
My largest advice for all builders is to maintain simplicity and group on the forefront as an increasing number of code turns into generated by AI.
Good Immediate, Unhealthy Immediate
When immediate directions are too imprecise you’ll almost certainly get {a partially} full reply containing directions on the way to fill in gaps. That is sometimes a lot much less helpful typically.
Listed here are just a few examples of prompts that lead to less-than-ideal code technology output:
Unhealthy Immediate
present code to make an api request to get my newest posts on reddit
i would like it to be proven on my web site as an inventory
Offering this instruction to a designer/developer is likely to be sufficient to make it a actuality the best way you’d count on, however not an AI immediate. It’s far too imprecise and lacks sufficient contextual data for AI to reliably create high quality code output.
- No point out of WordPress is supplied, so it’s seemingly that the code supplied gained’t be doable to make use of in your WP web site. It might be Python or server-side JavaScript.
- No context to work with the Reddit API is supplied, so its seemingly that you just gained’t get working code again and can as an alternative be given directions on the way to code it
- No particulars about the kind of content material to tug in from Reddit: feedback, posts or each? Particular subreddit or all subreddits?
- No particulars concerning the output you need, so it could present a advice for a plugin or software program that can be utilized as an alternative of code
In case your AI immediate does present PHP, which is unlikely however may occur relying on earlier conversations you’ve had, it nonetheless gained’t be code that’s all that helpful. It gained’t use any inside WP features like wp_remote_post
and wp_remote_retreive_body
and can as an alternative use uncooked PHP code to make requests with cURL.
The ensuing syntax will probably be more likely to fail in sure internet hosting environments, and also will be more durable for builders and immediate engineers to know and worth sooner or later.
As a result of there isn’t any context supplied concerning the request itself, no working code will probably be supplied. In the end it’s going to fall wanting expectations, and would require a superb quantity of follow-up to get nearer to a last product.
Whereas it’s doable and generally advantageous to make use of follow-up prompts whereas producing code, I like to recommend attempting to get as near last as doable with the primary immediate. Lengthy conversations with AI that commute typically lead to extra confusion, inaccuracy, and in the end frustration.
Good Immediate
Now for an instance of a significantly better immediate, that may lead to considerably higher outcomes.
create a wordpress perform that may make an API request utilizing wp_remote_post() to {REDDIT API URL} utilizing the API key {YOUR API-KEY} to generate an inventory of my most up-to-date posts and feedback. I at all times use my reddit deal with, {YOUR REDDIT-USERNAME}. the perform could have the next non-obligatory arguments:
- $restrict (integer) defaults to twenty, however accepts a quantity between 1 and 100. if an invalid worth is supplied return a WP_Error mentioning the difficulty
- $subreddits (array) defaults to [], accepts an array of strings containing particular subreddits to incorporate posts and feedback from. when supplied it's going to set the suitable API parameter(s), in any other case all posts and feedback are included
- $sort (string) defaults 'posts', however can be handed as 'feedback'. the worth of this parameter determines the kind of reddit content material we wish to checklist, posts or feedback, and can set the suitable API parameters/arguments to filter the returned outcomes
the response returned by wp_remote_post() will probably be checked for errors, and if a non-successful response was obtained again an in depth WP_Error will probably be returned with related details about the error.
any arguments handed to the perform will probably be validated for errors to confirm the codecs and kinds are right. when points are discovered a related WP_Error will probably be returned.
when a profitable 200 response is obtained again from the API request the response physique will probably be extracted with built-in WP core features (wp_remote_retreive_*) and restricted to professional ide a set of associative arrays, every one containing the next properties/keys:
- sort: remark|message
- topic: title/topic of the thread
- content material: both submit or remark textual content relying on the worth of sort, which may assist any HTML returned by the API
- published_on: ISO datetime when the content material was revealed
subreddit
- url: to both the submit or a hashed URL on to remark
this information will populate an HTML template based mostly on this Emmet construction:
div.reddit-feed>article.reddit-feed__item>h2.reddit-feed__title+p.reddit-feed__byline+div.reddit-feed__content
the template will:
- present a byline like this: "X days in the past at /r/{subreddit}" the place the subreddit is a hyperlink to the subreddit, and the times in the past makes use of the WP human time diff perform
- use wp_trim_words() to shorten any prolonged content material past 120 phrases, including a ellipses when shortened
- hyperlink the h2 title to both the remark or submit URL on reddit
- the __item could have a bem modifier figuring out the kind as both --post or --comment
additionally present SASS/SCSS code to type the template within the following methods:
- the highest degree container could have a high and backside margin between 20px and 40px, utilizing clamp() with a viewport width measurement rule to adapt based mostly on display screen widths between 600px and 1680px
- every merchandise could have a 20px backside margin and backside padding, with a 1.5px border-bottom that is 20% black
- the final merchandise could have no backside border, no padding and no margin
- the h2 could have a margin solely ln the underside of 10px, and will probably be 1.3x the font-size of the physique font-size
- the byline could have a margin solely on the underside of 20px, and will probably be 0.9x the font-size of the physique font-size. all textual content will probably be coloured 60% black, together with the subreddit hyperlink which will probably be recognized as a hyperlink with solely an underline
- the content material and any HTML inside it's going to haven't any margins
as soon as the ultimate code has been created evaluate it as an entire to determine any syntax or useful points to get it as near manufacturing prepared as doable
Superior Instance: Advanced Customized WP CLI Instructions
Placing all this into observe may look one thing like the next instance, which reveals the way to create a posh, detailed immediate to create a strong set of customized WP CLI instructions that may present methods to:
- Generate an search engine optimization report for your entire web site
- Safely create a brand new submit from a markdown file
- Discover and report any damaged hyperlinks inside submit content material and any ACF customized fields
To do that we have to present our AI immediate with many particular particulars, and it may be very useful to do that in a top level view format. When performed nicely, this may generate code at the least 10x quicker than it might sometimes take to put in writing from scratch.
It’s an amazing instance of how highly effective AI will be when used correctly for code technology, however it’s additionally fairly clear that to be actually efficient you do want a reasonably deep degree of information programming with WordPress as a way to point out particular features and approaches. Whilst you can generate code with out this information, the standard will probably be considerably higher, and fewer liable to errors when you do.
AI Immediate for WordPress Code Era
Create a PHP class for me so as to add into an current WordPress performance plugin.
- The namespace of the category will probably be Kevinlearynet
- The category identify will probably be
WP_CLI
- the category will use a singleton sample, and will probably be positioned in a single file when used
- It is going to add 3 customized WP CLI instructions to our WordPress web site:
- wp kevinlearynet create-post-from-markdown
- wp kevinlearynet list-seo-metadata
- wp kevinlearynet find-broken-links
- Every command will probably be a single class methodology that makes use of underscores and lowercase letters in its identify
- All instructions will present real-time output the place wanted to report on any profitable actions or errors as they happen
- All instructions will present a last abstract when full, or particulars about any errors which have occurred and why.
- Making a submit from markdown will:
- $title argument is non-obligatory, defaults to the H1 in markdown
- $markdown argument is required, if lacking or not legitimate markdown syntax an error message is supplied and the script is halted
- $slug argument is non-obligatory, defaults to slugified model of the title, utilizing the WP
sanitize_title()
perform - if an current submit is discovered with the identical slug or title an error message is supplied and the script is halted
- when a submit is efficiently created a URL to its edit view within the WP admin is supplied within the success output
- $standing argument is non-obligatory, defaults to draft however will be set to any legitimate post_status string
- Itemizing search engine optimization metadata command will:
- output will use the WP CLI desk format containing columns for submit title, url, date revealed, web optimization title, web optimization description
- use a customized
WP_Query
to get posts included within the output, with the next default parameters:- post_type is web page or submit
- posts_per_page is 500
- to web optimization title and web optimization description will probably be pulled from both the rank math or yoast web optimization plugin relying on which is put in and energetic. if neither is on the market an error message will probably be returned and the script will probably be halted
- settle for all the parameters obtainable to the built-in WP CLI submit checklist command will probably be allowed for filtering the
WP_Query
that selects posts included within the desk
- Discovering damaged hyperlinks command will:
- settle for all the parameters obtainable to the built-in WP CLI submit checklist command will probably be allowed for filtering the
WP_Query
that selects posts included within the desk - use a customized
WP_Query
to get posts included within the output, with the next default parameters:- post_type is web page or submit
- posts_per_page is -1
- discover all hyperlinks in the_content or any ACF customized fields for every submit returned by the customized question
- confirm all hyperlinks are legitimate and don’t return an error utilizing the built-in wp_http_get perform
- considers something not a 200 response to be damaged, even 301s and 302s
- when a number of damaged hyperlinks are discovered they are going to be an error message mentioning the variety of damaged hyperlinks discovered, and likewise a WP CLI desk format with columns for:
- hyperlink location (the content material or ACF discipline identify)
- url
- http response
- errors supplied throughout the scripts runtime is not going to halt or finish the script, they are going to solely be output and the script will proceed processing the subsequent submit
- when no damaged hyperlinks are discovered output the whole variety of efficiently examined hyperlinks
- output outcomes one after the other because the script runs
- settle for all the parameters obtainable to the built-in WP CLI submit checklist command will probably be allowed for filtering the
- as soon as full, check and confirm your entire class to substantiate there are not any errors and that the category is manufacturing prepared
- add a PHP docblock to the category containing:
- title: Customized WP CLI Instructions
- description: Provides customized CLI performance for quick publishing of markdown content material, speedy search engine optimization evaluation, and testing content material and customized fields for damaged hyperlinks
- add php docblock feedback to every methodology within the class, every with a title and quick, concise description of what it does
- use inline feedback sparingly inside all strategies
- embrace needed use statements for working inside the Kevinlearynet namespace
The Price of Straightforward
There’s little question about it, AI code technology makes programming quicker and accessible to extra folks. That is in some ways an excellent factor. If extra folks perceive how programming and code work, it’ll be simpler to work collectively and communicate the identical language. At the very least in idea.
I believe that the fact could also be totally different although. ChatGPT and different LLMs make it doable for folks to put in writing code that does issues with out actually understanding the way it works.
In some ways that is harmful, and it makes me consider the numerous poorly architected, frankenstein-like WordPress “purposes” I’ve inherited through the years. I’ve seen numerous websites hacked collectively in a make-it-work vogue that crumble in the long term.
It’s a basic case of saving cash upfront and paying dearly for it in the long term. This lesson will in all probability apply to AI code technology and lots of different areas as nicely. I may after all be means off right here, solely time will inform.
Conclusion
Whereas it’s harder and time-consuming to doc a immediate on this means, the rewards are nicely value it. The flexibility to put in writing high quality prompts that lead to exact, efficient generated code will probably be a essential talent for builders to have within the coming years.
Past this, it’s going to nonetheless be essential to be fluent within the language you’re working with and to take the time to know your codebase inside and outside.
AI code technology will proceed to revolutionize the best way web sites and purposes are constructed, and I believe codebases will broaden quickly consequently. Understanding the most effective methods to architect, construct, and in the end design good programs will probably be a talent that continues to be in demand for a while.