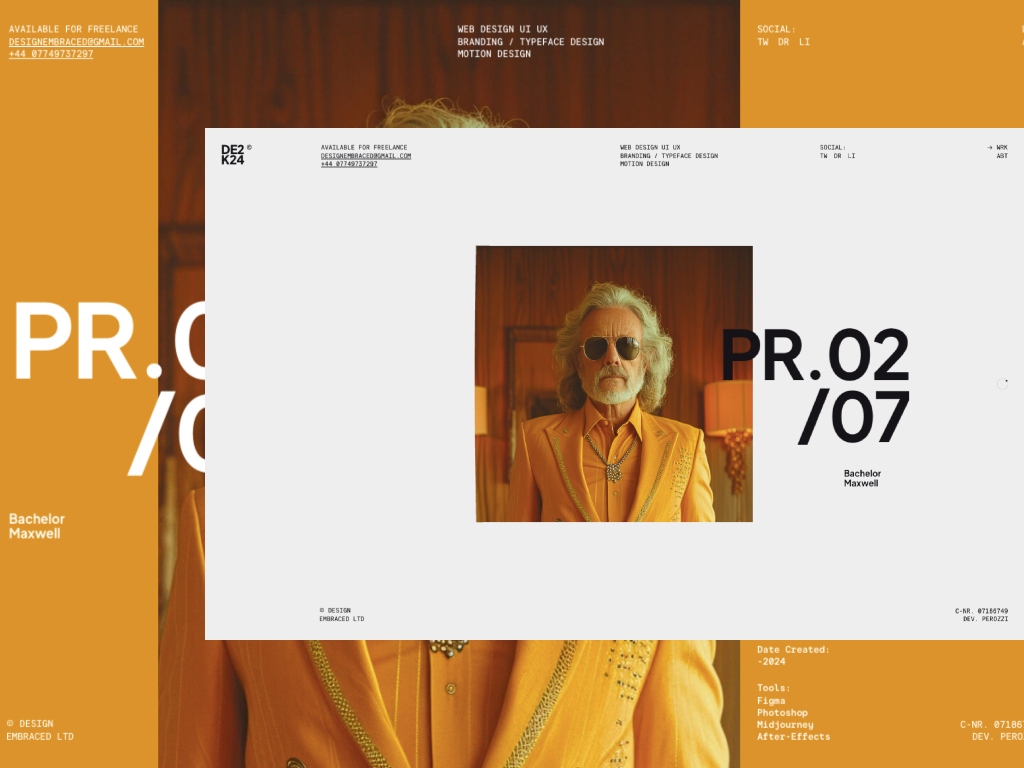
The way it began
Anthony obtained in contact with me via Norman. He had an idea prepared for his new portfolio and wanted somebody to deliver it to life. After he confirmed me this video of the house slider, I used to be on board instantly.
My first ideas
After agreeing to the collaboration, with out truly having a clue how one can do it, I assumed it might be easy to begin with the house slider and take care of the bending of the planes, since this impact could be used a number of instances. Including the required management factors to bend the aircraft correctly was simpler mentioned than executed. However, if I might try this, the remainder of the web page wouldn’t be so arduous anymore (a few web page transitions, some hover results, and many others.). No less than that’s what I assumed.
On this article
I’ll attempt to offer you some insights into the work I did with and for Anthony Goodwin, together with a few of my favourite options and animations. I can’t delve too deeply as a result of it might make the article fairly lengthy, and I don’t suppose it might be very fascinating, since most of it’s simply fundamental frontend stuff with a really distinctive movement idea and design that powers the entire expertise.
The tech stack
Earlier than beginning, I wished to outline the instruments and libraries to make use of for the web site. It’s mainly planes in 3D area with some vertex manipulation, requiring WebGL. Since we’re not importing any objects and all of the geometry is proscribed to planes, we don’t really want ThreeJS or another fancy (large) library. We are able to simply write it from scratch, which saves bytes and probably efficiency. Right here’s the tooling I ended up with:
The positioning doesn’t have a CMS, and the content material is editable via JSON. The main target was positively on the animation and the final really feel of the web page. Initially I deliberate on utilizing PayloadCMS (self-hosted). Test it out when you don’t comprehend it already. It’s top-of-the-line open supply CMS I got here throughout within the current years.
“Why not simply use NextJS, ThreeJS and GSAP? It’s simple!”
That’s a legitimate query. It in all probability would’ve been quicker, however a part of the method for me is studying new issues. I wasn’t actually acquainted with WebGL, GSAP, or the magic in NextJS (or Astro, for that matter). So, I made a decision to take the longer route to construct this challenge. It was extra about understanding the basics and the internal workings of every device than simply getting the challenge out the door. Additionally, I wished to enhance my very own “library” additional. It’s been a difficult but rewarding course of.
One other level price mentioning is that whereas these libraries are extremely helpful, however they’re additionally fairly massive as a result of they should cowl a variety of edge instances. Even with “tree-shaking” to take away unused code, I’d have used solely about 10%, making it overkill for this challenge. So I wished to go together with one thing extra light-weight.
Bending and curling planes
I’m unhealthy at math. That’s simply how it’s. So I want visualizations to make all of the capabilities tangible in any other case I wouldn’t be capable of provide you with something. Utilizing the Desmos Calculator, I used to be capable of create a graph that allowed me to manage the aircraft’s conduct the best way I wanted. It took me a while although. Right here’s the consequence, which you’ll be able to mess around with.
- s = The dimensions of the aircraft (or the size on this case)
- r1 = The radius of the curl
- k1 = The place of the curl
- u = Determines the course
There’re in all probability simpler and easier methods to resolve this. After I obtained the method down I attempted to use it to the planes vertex shader. Right here’s the consequence remoted:
And right here is the vertex shader code for the curl:
vec2 curlPlane(float x, float s, float r, float okay, bool flip) {
float v1 = flip ? s*okay : s - s*okay;
float n1 = s > 0.0 ? 1.0 : -1.0;
// Threshold earlier than going into the circle coords, as a result of
// if r is 0, it'll return infinity, and causes a brief
// flicker, so we stop that by setting a small
// non-noticable threshold
float t1 = 0.01;
// Begin and endpoints of the aircraft earlier than or after the curl
float e1 = flip ? n1*v1 : n1*x;
float e2 = flip ? n1*x : n1*v1;
// Some older gpus have troubles with "logical or operators"
// within the shader so we break up it into two circumstances as a substitute.
// Extra on that later within the article
if (r <= t1) {
return vec2(x, 0.0);
}
if (e1 <= e2) {
return vec2(x, 0.0);
}
float r2 = abs(s) / r;
float hp = 1.5707963;
// Rework the purpose on the aircraft to the purpose
// on the brand new arc related to the aircraft
return vec2(
v1/r2 + cos(x/r2 - hp - v1/r2),
-sin(x/r2 + hp - v1/r2) + 1.0
) * r2;
}
Web page Transitions
We aimed for seamless transitions between pages to reduce delay and keep a slick, simple navigation really feel. By preloading all of the pages upfront, we traded preliminary load efficiency for a greater person expertise and model identification. For web page transitions, I added animation performance to the router that works with the tween package deal.
I wished it to really feel much less like web page navigation and extra like one display altering states. Optimizing all animations to be interruptible was the toughest half. This additionally required that each mixture wanted an animation, so I ended up with all these completely different animations to cowl all instances:
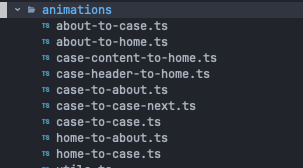
To get it proper with all of the scrolling and the states was the toughest a part of the portfolio to be trustworthy. Possibly there are higher methods to take care of this, however yeah creating a number of “sub-animations” as elements made it fairly manageable ultimately. Right here’s how the transition from “case to about” appears to be like:
import { RouterAnimation } from '@smoovy/router';
import { tween } from '@smoovy/tween';
import {
AnimationProps, enableTheme, isRoutePathMatch, sliderLeave, viewEnter,
viewLeave,
} from './utils';
export const caseToAbout = (props: AnimationProps) => ({
identify: 'caseToAbout',
when: ({ fromRoute, toRoute }) => {
return isRoutePathMatch(fromRoute, toRoute, '/case/', '/about');
},
enter: ({ toElement, fromElement }) => {
return tween.timeline({
onStart: () => enableTheme(props, toElement),
})
.add([
viewLeave(props, fromElement),
sliderLeave(props, fromElement)
])
.add(viewEnter(props, toElement), { offset: -.9 });
}
});
With out exhibiting an excessive amount of code right here, a fast description what’s behind these capabilities:
viewEnter
merely returns a timeline that triggers all of the animations on the incoming web page. Such because the textual content slide animation.viewLeave
additionally returns a timeline and triggers the “out” animation on all of those components in order that for instance the textual content slide up and out of view once more.sliderLeave
this hides the slider by curling the middle picture and shifting it to the highest, whereas additionally locking it and fading out all the opposite gadgets.enableTheme
prompts the colours discovered within the web page its navigating to when the transition begins
Utilizing animation util capabilities like sliderLeave
and viewEnter
allowed me to summary a few of the generic logic occurring on most pages, which made it simpler to debug. You’ll be able to check the web page transitions. They’re not excellent, but it surely positively feels extra fluid than simply having a fade-in fade-out transition. Right here’s an instance of me testing the interruptible transitions by fast navigation.
Shifting from case-to-case
We additionally determined to strive a seamless web page transition for looking the instances, working an animation and shortly hiding the previous web page.
To realize this transition I’m monitoring the scroll place from the beginning of the picture this.observable.y - this.viewport.peak * .5
to the tip this.observable.y
which is on the finish of the display. So when progress >= 1
and the transition hasn’t been triggered already, we navigate to the following challenge actually shortly.
/**
* this.observable.y is the place y of the following picture
* on the backside relative to the energetic web page.
*/
const finish = this.observable.y;
const begin = finish - this.viewport.peak * .5;
const progress = mapRange(scrollY, begin, finish, 0, 1);
/**
* Transfer the timeline for all view animations (textual content and many others.)
* with the scroll, so we get a pleasant scroll monitoring impact
*/
this.timeline.search(progress * this.timeline.period);
/**
* These values are used to calculate the scaling of the 1:1 picture
* to the brand new header picture of the following challenge. So we're simply aligning
* the dimensions of the "subsequent challenge picture" with the goal picture of the
* subsequent challenge. We additionally cross alongside the progress worth to the shader,
* so we are able to do that flip with the bend method from above!
*/
const scaleX = this.wrapper.width / this.imageTarget.width - 1;
const scaleY = this.viewport.peak / this.imageTarget.peak - 1;
for (const picture of this.photos) {
picture.scale({ x: 1 + scaleX * progress, y: 1 + scaleY * progress });
picture.uniforms.transition.worth = progress;
...
}
/**
* This merely triggers the router navigation when the progress is 1
* and the person has reached the tip of the location. We're then signaling
* the web page transition with the `subsequent` flag, that we wish to skip all
* animations on the picture and simply change instantenously. We additionally lock
* the scroller of the present web page so he cannot scroll again after the
* transition as been triggered.
*/
if (progress >= 1 && ! this.energetic && this.observable.seen) {
this.energetic = true;
this.scroller.lock();
this.router.to(this.url, { flags: { subsequent: true } });
}
My nemesis: Outdated GPU shader bug
I encountered a headache-inducing bug with the GPU in an older iMac, associated to using an or-operator in a situation contained in the shader. I needed to alter the shader code to work round this challenge.
So this didn’t work:
if (r <= t1 || e1 <= e2) {
return vec2(x, 0.0);
}
And needed to be reworked to:
if (r <= t1) {
return vec2(x, 0.0);
}
if (e1 <= e2) {
return vec2(x, 0.0);
}
If somebody has extra data on this, please let me know, since I couldn’t actually discover something helpful on-line, just a few previous stackoverflow threads with comparable points however not if that is meant conduct or actually a bug
The cellular web page
We opted for a non-WebGL model for cellular to maintain it light-weight and simplify improvement. Utilizing WebGL on cellular would require a loader and it’s higher for my part to make the cellular expertise as quick as doable with out a lot delay if doable. The cellular model replicates the house slider with CSS and JS, and web page transitions are simply easy fade-out and fade-in animations.
Making it accessible to keyboard-only utilization
Making certain accessibility for heavy-animation web sites is difficult. Even more durable when there’s a set price range and the primary focus is the model identification itself. For this portfolio, we’ve added keyboard navigation so customers can tab via all hyperlinks, the slider, and the instances. In an effort to mimic the default scrolling behaviour in browsers we added a “keyboard” conduct to the scroller:
Conclusion
Working with Anthony on his new portfolio taught me lots. I imply, he actually opened my eyes to lots. The entire course of was a mix of challenges, enjoyable, studying, lengthy nights and getting mad at some random GPU error. Collaborating with a artistic powerhouse like him was one thing else. He’s an absolute beast, and when you haven’t checked out his portfolio by now (which I doubt), go and take a look at his work! The most effective half was just about bouncing concepts round and experimentation. Nonetheless fascinated with how briskly Anthony is ready to translate concepts in his head to After Results. It saved us each plenty of forwards and backwards!
From a tech perspective, many devs will get it once I say I’d do issues otherwise in hindsight. However, ending the challenge and seeing individuals interact with it has been rewarding. Can’t await what’s subsequent!
When you’ve got any questions, be at liberty to ask me on Twitter or LinkedIn or attain out by way of mail!
P.S.: We received SOTD on 18th March on Awwwards, very glad about that! 🎉