Introduction
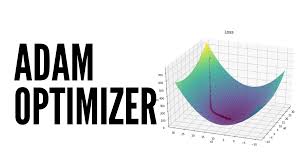
In deep studying, the Adam optimizer has develop into a go-to algorithm for a lot of practitioners. Its skill to adapt studying charges for various parameters and its light computational necessities make it a flexible and environment friendly selection. Nevertheless, Adam’s true potential lies within the fine-tuning of its hyperparameters. On this weblog, we’ll dive into the intricacies of the Adam optimizer in PyTorch, exploring tweak its settings to squeeze out each ounce of efficiency out of your neural community fashions.
Understanding Adam’s Core Parameters
Earlier than we begin tuning, it’s essential to grasp what we’re coping with. Adam stands for Adaptive Second Estimation, combining the perfect of two worlds: the per-parameter studying fee of AdaGrad and the momentum from RMSprop. The core parameters of Adam embody the educational fee (alpha), the decay charges for the primary (beta1) and second (beta2) second estimates, and epsilon, a small fixed to forestall division by zero. These parameters are the dials we’ll flip to optimize our neural community’s studying course of.
The Studying Price: Beginning Level of Tuning
The educational fee is arguably probably the most crucial hyperparameter. It determines the scale of our optimizer’s steps through the descent down the error gradient. A excessive fee can overshoot minima, whereas a low fee can result in painfully gradual convergence or getting caught in native minima. In PyTorch, setting the educational fee is easy:
optimizer = torch.optim.Adam(mannequin.parameters(), lr=0.001)
Nevertheless, discovering the candy spot requires experimentation and infrequently a studying fee scheduler to regulate the speed as coaching progresses.
Momentum Parameters: The Velocity and Stability Duo
Beta1 and beta2 management the decay charges of the shifting averages for the gradient and its sq., respectively. Beta1 is often set near 1, with a default of 0.9, permitting the optimizer to construct momentum and velocity up studying. Beta2, normally set to 0.999, stabilizes the educational by contemplating a wider window of previous gradients. Adjusting these values can result in sooner convergence or assist escape plateaus:
optimizer = torch.optim.Adam(mannequin.parameters(), lr=0.001, betas=(0.9, 0.999))
Epsilon: A Small Quantity with a Large Impression
Epsilon might sound insignificant, nevertheless it’s important for numerical stability, particularly when coping with small gradients. The default worth is normally adequate, however in instances of maximum precision or half-precision computations, tuning epsilon can forestall NaN errors:
optimizer = torch.optim.Adam(mannequin.parameters(), lr=0.001, eps=1e-08)
Weight Decay: The Regularization Guardian
Weight decay is a type of L2 regularization that may assist forestall overfitting by penalizing giant weights. In Adam, weight decay is utilized in another way, guaranteeing that the regularization is tailored together with the educational charges. This is usually a highly effective device to enhance generalization:
optimizer = torch.optim.Adam(mannequin.parameters(), lr=0.001, weight_decay=1e-4)
Amsgrad: A Variation on the Theme
Amsgrad is a variant of Adam that goals to resolve the convergence points through the use of the utmost of previous squared gradients quite than the exponential common. This may result in extra steady and constant convergence, particularly in advanced landscapes:
optimizer = torch.optim.Adam(mannequin.parameters(), lr=0.001, amsgrad=True)
Placing It All Collectively: A Tuning Technique
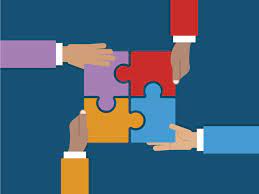
Tuning Adam’s parameters is an iterative course of that entails coaching, evaluating, and adjusting. Begin with the defaults, then modify the educational fee, adopted by beta1 and beta2. Regulate epsilon if you happen to’re working with half-precision, and think about weight decay for regularization. Use validation efficiency as your information; don’t be afraid to experiment.
Conclusion
Mastering the Adam optimizer in PyTorch is a mix of science and artwork. Understanding and thoroughly adjusting its hyperparameters can considerably improve your mannequin’s studying effectivity and efficiency. Keep in mind that there’s no one-size-fits-all answer; every mannequin and dataset might require a singular set of hyperparameters. Embrace the method of experimentation, and let the improved outcomes be your reward for the journey into the depths of Adam’s optimization capabilities.