Introduction
In Python programming, environment friendly information dealing with is paramount, and optimizing this course of is important for streamlined workflows. As you navigate the world of knowledge administration, one highly effective software is the Python Pickle moduleāa flexible resolution for object serialization. This module performs a vital position in preserving and storing Python objects, making certain their seamless retrieval and environment friendly dealing with, thereby contributing considerably to the general effectivity of knowledge operations.Ā
On this complete information, weāll navigate the intricacies of Python Pickle, unraveling its capabilities and understanding the way it facilitates seamless information serialization and deserialization. Whether or not youāre a seasoned developer or simply beginning with Python, this weblog will equip you with the information to harness the ability of Pickle in your tasks.
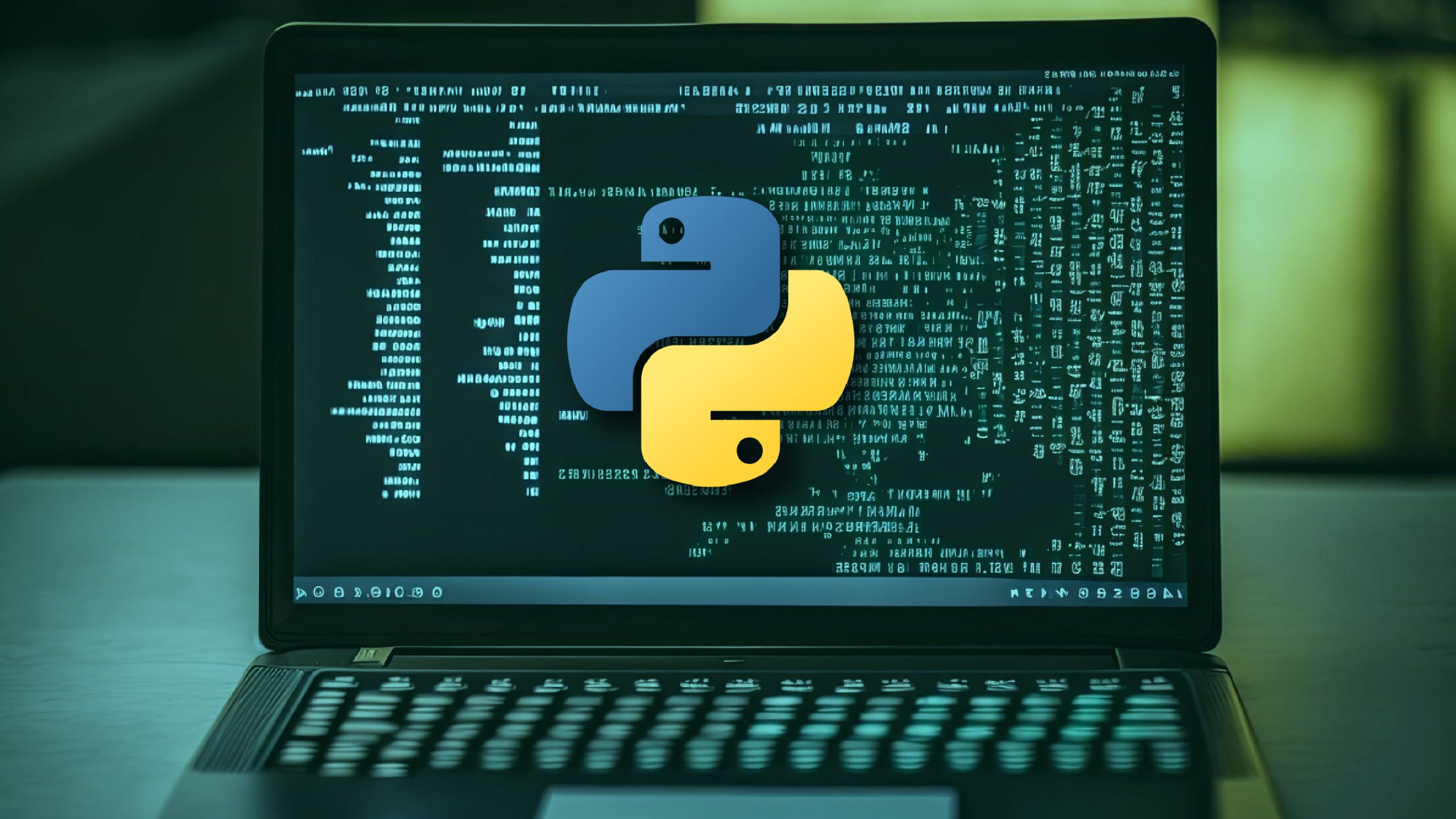
Understanding the Pickling Course of
In Python, the pickling course of includes changing an object right into a byte stream, which one can then retailer in a file or transmit over a community. The byte stream comprises all the data essential to reconstruct the article. When thereās a necessity to make use of the article once more, unpickling happens, changing the byte stream again into the unique object.
The Python Pickle module empowers us to serialize and deserialize Python objects. Serialization transforms an object right into a format appropriate for storage or transmission. Concurrently, deserialization is the reverse means of reconstructing the article from its serialized kind.
Why Use Python Pickle for Object Serialization?
Python Pickle presents a number of benefits in relation to object serialization.Ā
Firstly, it offers a easy and handy method to retailer and retrieve complicated information constructions. With Pickle, you possibly can simply save and cargo objects with out worrying in regards to the underlying particulars of the serialization course of.
Secondly, Pickle helps the serialization of virtually all built-in information varieties in Python, together with integers, floats, strings, lists, dictionaries, and extra. This makes it a flexible software for dealing with various kinds of information.
Lastly, Python Pickle lets you serialize customized objects, saving the state of your lessons and reusing them later. That is significantly helpful when working with machine studying fashions, the place it can save you and cargo the skilled mannequin for future predictions.
Python Pickle Strategies and Features
Pickle Module Overview
The Pickle module in Python offers a number of strategies and features for object serialization and deserialization. Letās take a more in-depth take a look at among the key ones:
Pickle.dump()
The `pickle.dump()` operate is used to serialize an object and write it to a file. It takes two arguments: the article to be serialized and the file object to which the serialized information shall be written.
Code
import pickle
information = {'identify': 'John', 'age': 30, 'metropolis': 'New York'}
with open('information.pickle', 'wb') as file:
Ā Ā Ā Ā pickle.dump(information, file)
Pickle.dumps()
The `pickle.dumps()` operate is just like `pickle.dump()`, however as a substitute of writing the serialized information to a file, it returns a byte string containing the serialized object.
Code
import pickle
information = {'identify': 'John', 'age': 30, 'metropolis': 'New York'}
serialized_data = pickle.dumps(information)
Pickle.load()
The `pickle.load()` operate deserializes an object from a file. It takes a file object as an argument and returns the deserialized object.
Code
import pickle
with open('information.pickle', 'rb') as file:
Ā Ā Ā Ā deserialized_data = pickle.load(file)
Pickle.masses()
The `pickle.masses()` operate is just like `pickle.load()`, however as a substitute of studying the serialized information from a file, it takes a byte string as an argument and returns the deserialized object.
Code
import pickle
serialized_data = b'x80x04x95x1bx00x00x00x00x00x00x00}x94(x8cx04namex94x8cx04Johnx94x8cx03agex94Kx1ex8cx04cityx94x8ctNew Yorkx94u.'
deserialized_data = pickle.masses(serialized_data)
Pickle.Pickler()
The `pickle.Pickler()` class customizes the pickling course of. It lets you outline your personal serialization logic for particular objects or information varieties.
Code
import pickle
class CustomPickler(pickle.Pickler):
Ā Ā Ā Ā def persistent_id(self, obj):
Ā Ā Ā Ā Ā Ā Ā Ā if isinstance(obj, MyCustomClass):
Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā return 'MyCustomClass', obj.id
Ā Ā Ā Ā Ā Ā Ā Ā return None
information = {'identify': 'John', 'age': 30, 'metropolis': 'New York'}
with open('information.pickle', 'wb') as file:
Ā Ā Ā Ā pickler = CustomPickler(file)
Ā Ā Ā Ā pickler.dump(information)
Pickle.Unpickler()
The `pickle.Unpickler()` class customizes the unpickling course of. It lets you outline your personal deserialization logic for particular objects or information varieties.
Code
import pickle
class CustomUnpickler(pickle.Unpickler):
Ā Ā Ā Ā def persistent_load(self, pid):
Ā Ā Ā Ā Ā Ā Ā Ā if pid[0] == 'MyCustomClass':
Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā return MyCustomClass(pid[1])
Ā Ā Ā Ā Ā Ā Ā Ā increase pickle.UnpicklingError(f"unsupported persistent object: {pid}")
with open('information.pickle', 'rb') as file:
Ā Ā Ā Ā unpickler = CustomUnpickler(file)
Ā Ā Ā Ā information = unpickler.load()
Working with Pickle in Python
Serializing Objects with Pickle
Pickle offers a handy method to serialize each built-in information varieties and customized objects. Letās discover find out how to use Pickle for object serialization.
Pickling Constructed-in Knowledge Sorts
Pickle helps serializing numerous built-in information varieties, comparable to integers, floats, strings, lists, dictionaries, and extra. Right hereās an instance of pickling a dictionary:
Code
import pickle
information = {'identify': 'John', 'age': 30, 'metropolis': 'New York'}
with open('information.pickle', 'wb') as file:
Ā Ā Ā Ā pickle.dump(information, file)
Pickling Customized Objects
Along with built-in information varieties, Pickle lets you serialize customized objects. To do that, the objects should be outlined in a module that may be imported. Right hereās an instance of pickling a customized object:
Code
import pickle
class Individual:
Ā Ā Ā Ā def __init__(self, identify, age):
Ā Ā Ā Ā Ā Ā Ā Ā self.identify = identify
Ā Ā Ā Ā Ā Ā Ā Ā self.age = age
particular person = Individual('John', 30)
with open('particular person.pickle', 'wb') as file:
Ā Ā Ā Ā pickle.dump(particular person, file)
Dealing with Pickle Errors and Exceptions
When working with Pickle, dealing with errors and exceptions could happen throughout the serialization or deserialization course of is essential. Widespread errors embrace `pickle.PickleError`, `pickle.PicklingError`, and `pickle.UnpicklingError`. Itās advisable to make use of try-except blocks to catch and deal with these errors appropriately.
Code
import pickle
strive:
Ā Ā Ā Ā with open('information.pickle', 'rb') as file:
Ā Ā Ā Ā Ā Ā Ā Ā information = pickle.load(file)
besides (pickle.PickleError, FileNotFoundError) as e:
Ā Ā Ā Ā print(f"Error occurred whereas unpickling: {e}")
Superior Pickling Strategies
Pickling and Inheritance
In Python, pickling and inheritance can typically result in sudden conduct. When a subclass is pickled, the superclass shouldn’t be mechanically pickled together with it. To make sure that the superclass can be pickled, you possibly can outline the `__getstate__()` and `__setstate__()` strategies within the subclass.
Code
import pickle
class Superclass:
Ā Ā Ā Ā def __init__(self, identify):
Ā Ā Ā Ā Ā Ā Ā Ā self.identify = identify
class Subclass(Superclass):
Ā Ā Ā Ā def __init__(self, identify, age):
Ā Ā Ā Ā Ā Ā Ā Ā tremendous().__init__(identify)
Ā Ā Ā Ā Ā Ā Ā Ā self.age = age
Ā Ā Ā Ā def __getstate__(self):
Ā Ā Ā Ā Ā Ā Ā Ā return self.identify, self.age
Ā Ā Ā Ā def __setstate__(self, state):
Ā Ā Ā Ā Ā Ā Ā Ā self.identify, self.age = state
subclass = Subclass('John', 30)
with open('subclass.pickle', 'wb') as file:
Ā Ā Ā Ā pickle.dump(subclass, file)
Pickling and Encapsulation
When pickling objects, itās essential to contemplate encapsulation. Pickling an object contains all its attributes, together with non-public and guarded ones. If you wish to exclude sure attributes from being pickled, you possibly can outline the `__getstate__()` methodology within the class and return a dictionary containing solely the specified attributes.
Code
import pickle
class Individual:
Ā Ā Ā Ā def __init__(self, identify, age):
Ā Ā Ā Ā Ā Ā Ā Ā self._name = identify
Ā Ā Ā Ā Ā Ā Ā Ā self._age = age
Ā Ā Ā Ā def __getstate__(self):
Ā Ā Ā Ā Ā Ā Ā Ā return {'identify': self._name}
Ā Ā Ā Ā def __setstate__(self, state):
Ā Ā Ā Ā Ā Ā Ā Ā self._name = state['name']
particular person = Individual('John', 30)
with open('particular person.pickle', 'wb') as file:
Ā Ā Ā Ā pickle.dump(particular person, file)
Pickling and Safety Issues
When utilizing Pickle, being conscious of potential safety dangers is essential. Pickle permits the execution of arbitrary code throughout the unpickling course of, which might result in code injection assaults. To mitigate this threat, itās advisable solely to unpickle information from trusted sources and keep away from unpickling untrusted information.
Greatest Practices and Suggestions for Utilizing Pickle
Pickle Efficiency Optimization
Protocol Choice
You possibly can choose the suitable protocol for serialization utilizing the `protocol` parameter of `pickle.dump()` or `pickle.dumps()`. Greater protocol variations usually lead to sooner serialization and smaller pickled recordsdata.
Code
import pickle
information = {'identify': 'John', 'age': 30, 'metropolis': 'New York'}
with open('information.pickle', 'wb') as file:
Ā Ā Ā Ā pickle.dump(information, file, protocol=pickle.HIGHEST_PROTOCOL)
Decreasing Pickle Dimension
Pickle recordsdata can typically be giant, particularly when serializing giant datasets. To cut back the scale of pickled recordsdata, you possibly can compress them utilizing the `gzip` module. This may considerably cut back the file measurement with out sacrificing the integrity of the information.
Code
import pickle
import gzip
information = {'identify': 'John', 'age': 30, 'metropolis': 'New York'}
with gzip.open('information.pickle.gz', 'wb') as file:
Ā Ā Ā Ā pickle.dump(information, file)
Dealing with Massive Datasets
Itās essential to contemplate reminiscence utilization and efficiency when working with giant datasets. As a substitute of pickling the whole dataset concurrently, you possibly can pickle it in smaller chunks or batches. This will help cut back reminiscence consumption and enhance total efficiency.
Code
import pickle
information = [...]Ā # Massive dataset
chunk_size = 1000
with open('information.pickle', 'wb') as file:
Ā Ā Ā Ā for i in vary(0, len(information), chunk_size):
Ā Ā Ā Ā Ā Ā Ā Ā chunk = information[i:i+chunk_size]
Ā Ā Ā Ā Ā Ā Ā Ā pickle.dump(chunk, file)
Pickle Compatibility and Versioning
Python Pickle helps versioning, which lets you deal with compatibility points when unpickling objects. By specifying a protocol model throughout pickling, you possibly can make sure that the pickled information might be efficiently unpickled even when the underlying class definitions have modified.
Code
import pickle
information = {'identify': 'John', 'age': 30, 'metropolis': 'New York'}
with open('information.pickle', 'wb') as file:
Ā Ā Ā Ā pickle.dump(information, file, protocol=2)
Pickle Options and Limitations
Whereas Python Pickle is a strong software for object serialization, it does have some limitations. Pickle is restricted to Python and can’t be used to serialize objects in different programming languages. Moreover, Pickle shouldn’t be safe in opposition to malicious assaults, so itās essential to train warning when unpickling untrusted information.
Potential Dangers and Safety Issues
Unpickling Untrusted Knowledge
One of many predominant safety considerations with Pickle is unpickling untrusted information. Since Pickle permits the execution of arbitrary code throughout the unpickling course of, it may be weak to code injection assaults. To mitigate this threat, solely unpickle information from trusted sources is essential.
Avoiding Pickle Bomb Assaults
A pickle bomb is a specifically crafted pickle object that may trigger a denial-of-service assault by consuming extreme system sources throughout unpickling. To stop pickle bomb assaults, we suggest limiting the utmost measurement of the pickled information utilizing the sys.setrecursionlimit() operate.
Code
import sys
import pickle
sys.setrecursionlimit(10000)
information = [...]Ā # Massive dataset
with open('information.pickle', 'wb') as file:
Ā Ā Ā Ā pickle.dump(information, file)
Safe Pickling Practices
To make sure safe pickling, itās essential to observe some greatest practices. Firstly, solely unpickle information from trusted sources. Secondly, keep away from pickling untrusted information or information that will include malicious code. Lastly, often replace your Python model and the modules you employ to profit from the newest safety patches.
Conclusion
Python Pickle is a strong module for object serialization in Python. It offers a easy and handy method to retailer and retrieve complicated information constructions, helps serializing built-in information varieties and customized objects, and presents numerous superior strategies for pickling and unpickling. Nonetheless, itās essential to pay attention to the potential dangers and safety considerations related to Pickle and observe greatest practices to make sure safe pickling. By understanding and using the capabilities of Python Pickle, you possibly can successfully serialize and deserialize objects in your Python purposes.
Grasp Python for Knowledge Science with our Licensed AI & ML BlackBelt Plus Program. Elevate your expertise from primary to superior, solidify coding experience, and construct impactful tasks. Achieve mentorship for Python interviews and obtain a certification from Analytics Vidhya. Begin your Python studying journey immediately!