Python lists are versatile knowledge buildings that permit us to retailer and manipulate collections of things. Some of the highly effective options of lists is the power to entry and modify particular person parts utilizing their index values. On this article, we’ll discover varied strategies and strategies for working with record indices in Python.
Understanding the Python Checklist Index
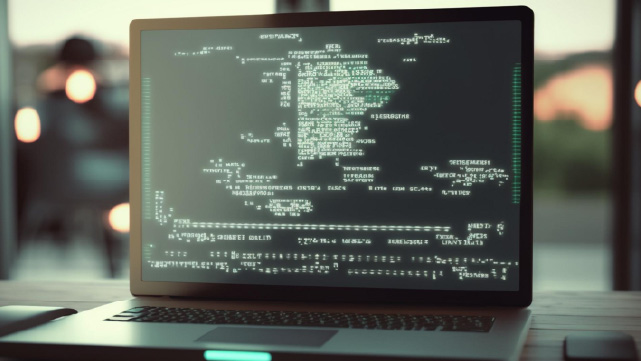
The index of a component in a listing refers to its place throughout the record. The primary aspect has an index of 0, the second aspect has an index of 1, and so forth. We are able to retrieve or modify particular parts in a listing through the use of the index.
We are able to use the sq. bracket notation to entry a component at a selected index. For instance, if we have now a listing known as `my_list` and we wish to entry the aspect at index 2, we will use the next code:
Code:
my_list = [10, 20, 30, 40, 50]
aspect = my_list[2]
print(aspect)
Output:
30
On this instance, `my_list[2]` returns the aspect at index 2, which is 30.
Superior Methods for Python Checklist Indexing
Discovering the First Incidence of an Component
Typically, we might have to seek out the index of the primary prevalence of a selected aspect in a listing. We are able to obtain this through the use of the `index()` technique. As an example, if we have now a listing known as `fruits` and we wish to discover the index of the primary prevalence of the aspect “apple”, we will use the next code:
Code
fruits = ["apple", "banana", "orange", "apple", "mango"]
index = fruits.index("apple")
print(index)
Output:
0
On this instance, the `fruits.index(“apple”)` returns the index of the primary prevalence of “apple”, which is 0.
Discovering All Occurrences of an Component
We are able to mix record comprehension and the `enumerate()` operate to seek out all occurrences of a selected aspect in a listing. For instance, if we have now a listing known as `numbers` and we wish to discover all indices of the aspect 5, we will use the next code:
Code:
numbers = [1, 5, 2, 5, 3, 5]
indices = [index for index, value in enumerate(numbers) if value == 5]
print(indices)
Output:
[1, 3, 5]
On this instance, the record comprehension `[index for index, value in enumerate(numbers) if value == 5]` generates a brand new record containing the indices of all occurrences of aspect 5.
Dealing with Nonexistent Parts
When attempting to entry a component at an index that’s out of vary, Python raises an `IndexError` exception. To deal with this case, we will use a try-except block. As an example, if we have now a listing known as `my_list` with three parts and we attempt to entry the aspect at index 5, we will use the next code:
Code:
my_list = [10, 20, 30]
attempt:
aspect = my_list[5]
print(aspect)
besides IndexError:
print("Index out of vary")
Output:
Index out of vary
On this instance, since index 5 is out of vary for `my_list`, the code contained in the besides block might be executed, and the message “Index out of vary” might be printed.
Utilizing Adverse Indices
Along with optimistic indices, Python additionally helps destructive indices. Adverse indices depend from the top of the record, with -1 representing the final aspect, -2 representing the second-to-last aspect, and so forth. This may be helpful once we wish to entry parts from the top of the record. For instance, if we have now a listing known as `my_list` and we wish to entry the final aspect, we will use the next code:
Code:
my_list = [10, 20, 30, 40, 50]
aspect = my_list[-1]
print(aspect)
Output:
50
On this instance, `my_list[-1]` returns the final aspect of the record, which is 50.
Looking out inside Sublists
Typically, we could have a listing of lists and wish to seek for a selected aspect throughout the sublists. We are able to obtain this through the use of nested loops and record indices. As an example, if we have now a listing known as `matrix` and we wish to discover the indices of all occurrences of the aspect 0, we will use the next code:
Code:
matrix = [[1, 2, 3], [4, 0, 6], [7, 8, 9]]
indices = [(row_index, col_index) for row_index, row in enumerate(matrix) for col_index, value in enumerate(row) if value == 0]
print(indices)
Output:
[(1, 1)]
On this instance, the record comprehension generates a brand new record containing the indices of all occurrences of the aspect 0 throughout the sublists.
Checklist Indexing with Constructed-in Capabilities and Libraries
Using the ‘in’ Operator
The `in` operator can test if a component exists in a listing. It returns a boolean worth indicating whether or not the aspect is current or not. For instance, if we have now a listing known as `my_list` and we wish to test if the aspect 10 is current, we will use the next code:
Code:
my_list = [10, 20, 30, 40, 50]
if 10 in my_list:
print("Component discovered")
else:
print("Component not discovered")
Output:
Component discovered
On this instance, since aspect ten is current in `my_list`, the message “Component discovered” might be printed.
Utilizing the ‘index’ Perform
The `index()` operate is a built-in technique in Python that returns the index of the primary prevalence of a specified aspect in a listing. For instance, if we have now a listing known as `numbers` and we wish to discover the index of the primary prevalence of the aspect 5, we will use the next code:
Code:
numbers = [1, 5, 2, 5, 3, 5]
index = numbers.index(5)
print(index)
Output:
1
On this instance, `numbers.index(5)` returns the index of the primary prevalence of 5, which is 1.
Exploring the ‘numpy’ Library
The ‘numpy’ Library is a strong software for scientific computing in Python. It offers environment friendly knowledge buildings and capabilities for arrays, together with indexing operations. For instance, if we have now a numpy array known as `my_array` and we wish to entry the aspect at index (2, 3), we will use the next code:
Code:
import numpy as np
my_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
aspect = my_array[2, 2]
print(aspect)
Output:
9
On this instance, `my_array[2, 2]` returns the aspect at index (2, 2), which is 9.
Leveraging the ‘Pandas’ Library
The ‘pandas’ Library is a well-liked Python knowledge manipulation and evaluation software. It offers highly effective knowledge buildings just like the DataFrame, permitting environment friendly indexing and slicing operations. For instance, if we have now a DataFrame known as `df` and we wish to entry the worth within the “age” column at index 2, we will use the next code:
Code:
import pandas as pd
knowledge = {'identify': ['Nishu', 'Tarun', 'Himanshu'],
'age': [25, 30, 35]}
df = pd.DataFrame(knowledge)
worth = df.at[2, 'age']
print(worth)
Output:
35
On this instance, `df.at[2, ‘age’]` returns the worth within the “age” column at index 2, which is 35.
Sensible Examples and Use Instances
Discovering the Index of the Most or Minimal Component
To seek out the index of the utmost or minimal aspect in a listing, we will use the `index()` operate together with the `max()` or `min()` operate. For instance, if we have now a listing known as `numbers` and we wish to discover the index of the utmost aspect, we will use the next code:
Code:
numbers = [10, 5, 20, 15, 30]
max_index = numbers.index(max(numbers))
print(max_index)
Output:
4
On this instance, `numbers.index(max(numbers))` returns the index of the utmost aspect, which is 4.
Trying to find Particular Patterns or Substrings
We are able to use record comprehension and string strategies to seek for particular patterns or substrings inside a listing. For instance, if we have now a listing known as `phrases` and we wish to discover all indices of phrases that begin with the letter “a”, we will use the next code:
Code:
phrases = ["apple", "banana", "avocado", "orange"]
indices = [index for index in enumerate(words) if word.startswith("a")]
print(indices)
Output:
[0, 2]
On this instance, the record comprehension `[index for index, word in enumerate(words) if word.startswith(“a”)]` generates a brand new record containing the indices of all phrases that begin with “a”.
Reversing a Checklist Utilizing Indexing
We are able to use record slicing with a step worth of -1 to reverse the order of parts in a listing. For instance, if we have now a listing known as `my_list` and we wish to reverse it, we will use the next code:
Code:
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1]
print(reversed_list)
Output:
[5, 4, 3, 2, 1]
On this instance, `my_list[::-1]` returns a brand new record with the weather in reverse order.
Eradicating Duplicates from a Checklist
We are able to mix record comprehension and the’ not in’ operator to take away duplicates from a listing whereas preserving the order of parts. For instance, if we have now a listing known as `my_list` with duplicate parts and we wish to take away them, we will use the next code:
Code:
my_list = [1, 2, 3, 2, 4, 5, 1]
unique_list = [x for i, x in enumerate(my_list) if x not in my_list[:i]]
print(unique_list)
Output:
[1, 2, 3, 4, 5]
On this instance, the record comprehension `[x for i, x in enumerate(my_list) if x not in my_list[:i]]` generates a brand new record containing solely the distinctive parts.
Sorting a Checklist Based mostly on Index Values
To kind a listing based mostly on the index values of its parts, we will use the `sorted()` operate with a customized key operate. For instance, if we have now a listing known as `fruits` and we wish to kind it based mostly on the index values of the weather, we will use the next code:
Code:
fruits = ["banana", "apple", "orange", "mango"]
sorted_fruits = sorted(fruits, key=lambda x: fruits.index(x))
print(sorted_fruits)
Output:
[“banana”, “apple”, “orange”, “mango”]
On this instance, `sorted(fruits, key=lambda x: fruits.index(x))` returns a brand new record with the weather sorted based mostly on their index values.
Ideas for Environment friendly Python Checklist Indexing
When working with lists in Python, environment friendly indexing is essential for optimizing efficiency and reminiscence utilization. On this part, we’ll discover some suggestions and strategies to boost the effectivity of record indexing.
Utilizing Checklist Comprehension
Checklist comprehension is a strong characteristic in Python that enables us to create new lists by iterating over an current record and making use of sure situations or transformations. It may also be used for environment friendly indexing.
For instance, suppose we have now a listing of numbers and wish to create a brand new record containing solely the even numbers. As a substitute of utilizing a conventional for loop, we will use record comprehension to realize this extra concisely and effectively:
Code:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers)
Output:
[2, 4, 6, 8, 10]
On this instance, the record comprehension `[num for num in numbers if num % 2 == 0]` filters out the odd numbers from the unique record and creates a brand new record containing solely the even numbers. This strategy reduces the quantity of code and improves the effectivity of indexing.
Using Binary Seek for Massive Lists
Binary search is a generally used algorithm for looking parts in a sorted record. It’s helpful when coping with giant lists, because it has a time complexity of O(log n) in comparison with the linear time complexity of O(n) for sequential search.
The record should be sorted in ascending order to make use of binary seek for environment friendly record indexing. We are able to then use Python’s `bisect` module to carry out a binary search.
Code:
import bisect
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
index = bisect.bisect_left(numbers, 5)
print(index)
Output:
4
On this instance, `bisect.bisect_left(numbers, 5)` returns the index of the leftmost prevalence of the quantity 5 within the sorted record. If the quantity isn’t discovered, it returns the index the place the quantity needs to be inserted to take care of the sorted order.
Through the use of binary search, we will considerably cut back the time complexity of indexing giant lists, making our code extra environment friendly.
Contemplating Time Complexity
When performing record indexing operations, it’s important to contemplate the time complexity of the operations concerned. Totally different operations have completely different time complexities, and selecting probably the most environment friendly operation can considerably affect the efficiency of our code.
For instance, accessing a component by its index has a time complexity of O(1), because it straight retrieves the aspect on the specified index. However, looking for a part utilizing the `index()` technique has a time complexity of O(n), because it must iterate by means of the complete record to seek out the aspect.
Subsequently, if we have to carry out frequent index-based operations, it’s advisable to make use of direct indexing as an alternative of looking for parts. This will considerably enhance the effectivity of our code.
Optimizing Reminiscence Utilization
Along with optimizing time complexity, optimizing reminiscence utilization when working with lists can be important. This might help cut back the general reminiscence footprint of our program and enhance its efficiency.
One strategy to optimize reminiscence utilization is utilizing generator expressions as an alternative of making intermediate lists. Generator expressions are much like record comprehensions however return an iterator as an alternative of a listing. This implies they devour much less reminiscence as they generate parts on the fly.
Code:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = (num for num in numbers if num % 2 == 0)
print(even_numbers)
Output:
<generator object <genexpr> at 0x7d63bf832490>
On this instance, `(num for num in numbers if num % 2 == 0)` is a generator expression that generates solely the even numbers from the unique record. We are able to save reminiscence when working with giant lists through the use of a generator expression as an alternative of a listing comprehension.
Exploring Different Knowledge Buildings
Whereas lists are a flexible knowledge construction in Python, they might not at all times be probably the most environment friendly selection for sure use circumstances. Relying on the precise necessities of our program, it could be price exploring various knowledge buildings that supply higher efficiency for indexing operations.
For instance, if we have to carry out frequent insertions or deletions at each ends of the record, a deque (double-ended queue) from the `collections` module could also be a more sensible choice. Deques present environment friendly O(1) time complexity for these operations.
Alternatively, a set or a dictionary could also be extra environment friendly if we have to carry out frequent membership exams (checking if a component is current within the record). Units and dictionaries have a median time complexity of O(1) for membership exams in comparison with O(n) for lists.
By contemplating the precise necessities of our program and exploring various knowledge buildings, we will select probably the most environment friendly knowledge construction for our indexing wants.
Conclusion
An environment friendly Python record index is crucial for optimizing our packages’ efficiency and reminiscence utilization. By following the ideas and strategies mentioned on this article, we will improve the effectivity of our record indexing operations. We explored record comprehension for concise and environment friendly indexing, employed binary seek for giant lists, thought of time complexity for optimum efficiency, optimized reminiscence utilization with generator expressions, and explored various knowledge buildings for particular use circumstances. By making use of these practices, we will write extra environment friendly and sturdy code when working with record indexing in Python.