Introduction
In September 2023, the Mistral Lab launched Mistral-7b, a completely open-sourced mannequin with an Apache 2.0 license. It took the AI sphere by storm and topped the Open LLM leaderboard. It outperformed greater fashions like Llama 2 13b on all benchmarks. Even now, the fashions topping the leaderboard are derived from the Mistral base mannequin. It has been confirmed that Mistral-7b is a succesful mannequin and has a ton of potential. Nonetheless, for a lot of duties, a base mannequin won’t be fascinating. The fashions normally wanted to be skilled over customized datasets to carry out higher at focused duties like Coding, Function-Play, Chat, and so forth. In addition to these fashions, even the 7B parameter could be very costly to fine-tune. Totally fine-tuning these fashions requires important quantities of GPUs. However because of latest developments in mannequin quantization and LoRA, we are able to now fine-tune and infer from small LLMs at no cost.
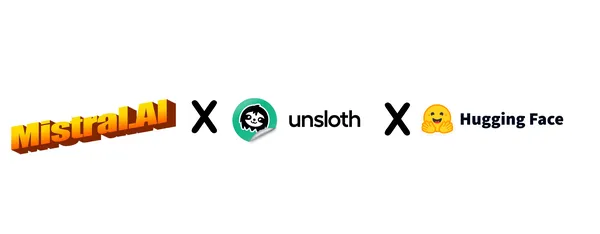
Studying Targets
- Study LLM fine-tuning.
- Perceive the fundamentals of LoRA and QLoRA.
- Discover instruments and strategies for fine-tuning.
- Implement SFT fine-tuning of Mistral-7b on the Colab utilizing Unsloth and HuggingFace’s trl library.
This text was revealed as part of the Information Science Blogathon.
High-quality-tuning LLMs
High-quality-tuning is one of the simplest ways to make a mannequin find out about task-specific issues. It’s the course of of coaching a pre-trained mannequin over customized datasets to make the mannequin carry out higher on focused duties. Throughout fine-tuning, the parameters of the bottom mannequin are up to date by means of switch studying to replicate the information realized.
All of the mannequin parameters are up to date throughout full fine-tuning, however this may be very costly and inaccessible to a bigger chunk of builders. That is the place LoRA and QLoRA come into the image. So, let’s perceive LoRA and QLoRA.
LoRA
LoRA (Low-Rank Adaptation) is an environment friendly methodology for fine-tuning language fashions with fewer computing assets by decreasing the variety of trainable parameters. The LoRA relies on the low-rank approximation method to approximate a big matrix as carefully as doable.
Observe: A rank of a matrix is the variety of linearly unbiased rows or columns. That is analogous to dimensionality discount. SVD(Singular Worth Decomposition) and PCA(Principal Part Evaluation) are used for low-rank decomposition of authentic weight matrices.
In distinction to full fine-tuning, the place all of the weights are up to date, with LoRA, solely the low-rank approximated weight matrices are up to date; these are referred to as replace matrices. This can be a important enchancment in velocity and effectivity over full fine-tuning, as we’ll solely cope with a low-rank matrix of the unique weight matrix.
The unique parameters stay the identical because the LoRA, however solely low-rank matrices (adapters) are up to date. This reduces the general GPU necessities for fine-tuning. These adapters act as add-ons at the side of authentic weights for token prediction.
QLoRA
The LoRA was an amazing step up over full fine-tuning, but it was nonetheless costly to fine-tune fashions on consumer-grade machines. That is the place QLoRA shines.
The QLoRA stands for Quantized LoRA. Quantization is casting high-bit numbers to low-bit numbers to cut back reminiscence footprint. Authentic fashions normally have increased bit-values (float16, 32) to retailer extra data. However it additionally requires giant computing assets to work with them.
To make the method environment friendly, QLoRA launched three totally different ideas. They’re 4-bit Regular Float 16(NF4), optimum for usually distributed weights, Double Quantization to cut back common reminiscence footprint by quantizing the quantization fixed, and Paged Optimizer to cut back reminiscence spikes.
As soon as the quantization is full, the LoRA is utilized to fine-tune low-rank replace matrices. Your entire means of mannequin quantization adopted by LoRA makes it straightforward to fine-tune LLMs on client {hardware}.
Additionally Learn: What’s QLoRA?
High-quality-tuning with Unsloth
Unsloth is an open-source platform for environment friendly fine-tuning of in style open-source LLMs like Llama-2, Mistral, and different derivatives. Unsloth implements optimized Triton kernels, guide autograds, and so forth, to hurry up coaching. It’s virtually twice as quick as Huggingface and Flash Consideration implementation.
We are going to use Unsloth to fine-tune a Mistral-7b mannequin on the Alpaca dataset over Colab’s free Tesla T4 GPU.
First, open a Colab pocket book with a GPU runtime and set up the next libraries.
import torch
!pip set up "unsloth[colab] @ git+https://github.com/unslothai/unsloth.git"
!pip set up "git+https://github.com/huggingface/transformers.git"123python
Now, we obtain the 4-bit Mistral 7b mannequin to our runtime by means of Unsloth’s FastLanguageModel class.
from unsloth import FastLanguageModel
import torch
max_seq_length = 2048
dtype = None # None for auto detection. Float16 for Tesla T4, V100, Bfloat16 for Ampere+
load_in_4bit = True # Use 4bit quantization to cut back reminiscence utilization. May be False.
mannequin, tokenizer = FastLanguageModel.from_pretrained(
model_name = "unsloth/mistral-7b-bnb-4bit", # "unsloth/mistral-7b" for 16bit loading
max_seq_length = max_seq_length,
dtype = dtype,
load_in_4bit = load_in_4bit,
# token = "hf_...", # use one if utilizing gated fashions like meta-llama/Llama-2-7b-hf
)
To make use of 16-bit fashions, set load_in_4bit to False.
Now, add LoRA adapters. So, we solely must cope with a fraction of parameters (1-10%).
mannequin = FastLanguageModel.get_peft_model(
mannequin,
r = 16, # Select any quantity > 0 ! Urged 8, 16, 32, 64, 128
target_modules = ["q_proj", "k_proj", "v_proj", "o_proj",
"gate_proj", "up_proj", "down_proj",],
lora_alpha = 16,
lora_dropout = 0, # At present solely helps dropout = 0
bias = "none", # At present solely helps bias = "none"
use_gradient_checkpointing = True,
random_state = 3407,
max_seq_length = max_seq_length,
)
Information Preparation
We are going to use the Yahma model of the Alpaca 52k dataset. This can be a cleaned model of the unique Alpaca dataset from Stanford. The dataset has information in instruction-output format. Right here is an instance

Now, put together the dataset for fine-tuning by loading the dataset from the HuggingFace datasets library.
alpaca_prompt = """Under is an instruction that describes a process,
paired with an enter that gives additional context. Write a response that
appropriately completes the request.
### Instruction:
{}
### Enter:
{}
### Response:
{}"""
def formatting_prompts_func(examples):
directions = examples["instruction"]
inputs = examples["input"]
outputs = examples["output"]
texts = []
for instruction, enter, output in zip(directions, inputs, outputs):
textual content = alpaca_prompt.format(instruction, enter, output)
texts.append(textual content)
return { "textual content" : texts}
move
from datasets import load_dataset
dataset = load_dataset("yahma/alpaca-cleaned", cut up = "prepare")
dataset = dataset.map(formatting_prompts_func, batched = True,)
Coaching the Mannequin
To date, now we have loaded a 4-bit quantized Mistral-7b mannequin, created a LoRA configuration, and ready our information for coaching. The subsequent factor is to coach the mannequin. There are a number of methods to perform mannequin coaching, akin to SFT and DPO. Let’s briefly undergo these ideas.
SFT
SFT stands for Supervised High-quality Tuning, and because the title suggests in SFT, we may have a labeled dataset much like the Alpaca dataset we simply ready. The dataset consists of information with directions and anticipated solutions. The fashions fine-tuned over it study the sample and nuances of anticipated solutions related to questions.
DPO
DPO stands for Direct Desire Optimisation. The dataset for DPO consists of information with directions, an accepted reply, and a rejected reply. Right here is an instance of a DPO dataset.
DPO approaches the duty as a classification downside. To perform this, it employs two fashions: A skilled mannequin and a reference mannequin. Throughout fine-tuning, the purpose is to make the skilled mannequin yield increased chances for accepted responses than the reference mannequin. Conversely, we will even need the coverage mannequin to output decrease chances for rejected solutions than the reward mannequin.
We will effectively align mannequin conduct with our desire by rewarding the mannequin for most popular responses and penalizing it for rejected responses.
Now, again to our mannequin coaching. We are going to use the Supervised High-quality Tuning (SFT) methodology to coach the LoRA adapters on the Alpaca dataset. To perform this, we’ll use the SFTTrainer from the TRL library. For DPO, there’s a DPOTrainer class.
from trl import SFTTrainer
from transformers import TrainingArguments
coach = SFTTrainer(
mannequin = mannequin,
train_dataset = dataset,
dataset_text_field = "textual content",
max_seq_length = max_seq_length,
args = TrainingArguments(
per_device_train_batch_size = 2,
gradient_accumulation_steps = 4,
warmup_steps = 5,
max_steps = 60,
learning_rate = 2e-4,
fp16 = not torch.cuda.is_bf16_supported(),
bf16 = torch.cuda.is_bf16_supported(),
logging_steps = 1,
optim = "adamw_8bit",
weight_decay = 0.01,
lr_scheduler_type = "linear",
seed = 3407,
output_dir = "outputs",
),
)
Now, begin the coaching.
trainer_stats = coach.prepare()
This can take some time. As soon as the coaching is completed, we are able to use the fine-tuned mannequin for inferencing.
Inference
Now, run the mannequin with formatted inputs.
inputs = tokenizer(
[
alpaca_prompt.format(
"Continue the fibonnaci sequence.", # instruction
"1, 1, 2, 3, 5, 8", # input
"", # output - leave this blank for generation!
)
]*1, return_tensors = "pt").to("cuda")
outputs = mannequin.generate(**inputs, max_new_tokens = 128, use_cache = True)
tokenizer.batch_decode(outputs)
This can output a listing with a string of the mannequin’s directions, inputs, and outputs.

We will save the LoRA adapters to the native listing with the next code.
mannequin.save_pretrained("mistral_lora_model")
If you happen to want to push the mannequin to Huggingface Hub, create a HugginFace account and run the beneath code
mannequin.push_to_hub("your_name/mistral_lora_model")
We will load the saved LoRA adapters and inference as nicely.
from peft import PeftModel
mannequin = PeftModel.from_pretrained(mannequin, "mistral_lora_model")
Outline a perform to format and print the mannequin output.
from typing import Record
def get_response(question:str, enter="")->Record[str]:
inputs = tokenizer(
[
alpaca_prompt.format(
query, # instruction
input, # input
"", # output
)
]*1, return_tensors = "pt").to("cuda")
outputs = mannequin.generate(**inputs, max_new_tokens = 1024, use_cache = True)
return tokenizer.batch_decode(outputs)
question = "State 3 distinctive features of the next quantity?"
enter = "1729"
resp = get_response(question, enter)
def format_msg(message):
split_msg = message.cut up("### ")
final_str = split_msg[1]+split_msg[3]
return final_str
print(format_msg(resp[0]))

You may play with the prompts and see the way it performs.
Conclusion
The LoRA and QLoRA have made operating LLMs on client {hardware} a actuality with out sacrificing the unique efficiency of the fashions. A real democratization of Giant Language Fashions. With the assistance of instruments like Unsloth, transformers, and trl, it’s doable to fine-tune LLMs on customized datasets over client GPUs. This text confirmed the best way to fine-tune an LLM in Colab’s T4 GPU.
Key Takeaways
- High-quality-tuning is one of the simplest ways to make a mannequin obey particular directions. It makes fashions study patterns from smaller datasets.
- Whereas full fine-tuning is at all times desired, the mannequin coaching price could be costly for customized use circumstances.
- LoRA simplifies this by solely needing us to coach low-rank replace matrices as an alternative of full-weight matrices.
- Whereas LoRA is a step up, the QLoRA makes it much more cost-effective by quantizing fashions earlier than making use of LoRA.
- Unsloth is an open-source platform that gives instruments to hurry up fine-tuning LLMs.
Continuously Requested Questions
A. Mistral-7b is a completely open-source Giant Language Mannequin from Mistral lab with a wonderful potential to fine-tune over customized datasets.
A. It’s doable to fine-tune smaller LLMs at no cost on Colab over the Tesla T4 GPU with QLoRA.
A. High-quality-tuning vastly enhances LLM’s functionality to carry out downstream duties, like function play, code era, and so forth.
A. The LoRA is a fine-tuning methodology the place solely a fraction of approximated mannequin weights are skilled as an alternative of the unique weights. Thus decreasing the general reminiscence footprint. Whereas in QLoRA, the fashions are quantized earlier than making use of LoRA. This makes fine-tuning much less GPU-intensive.
A. High-quality-tuning has many benefits however may skew the mannequin conduct by introducing biases. The fine-tuning information have to be completely examined earlier than coaching the mannequin on it.
The media proven on this article shouldn’t be owned by Analytics Vidhya and is used on the Creator’s discretion.Â