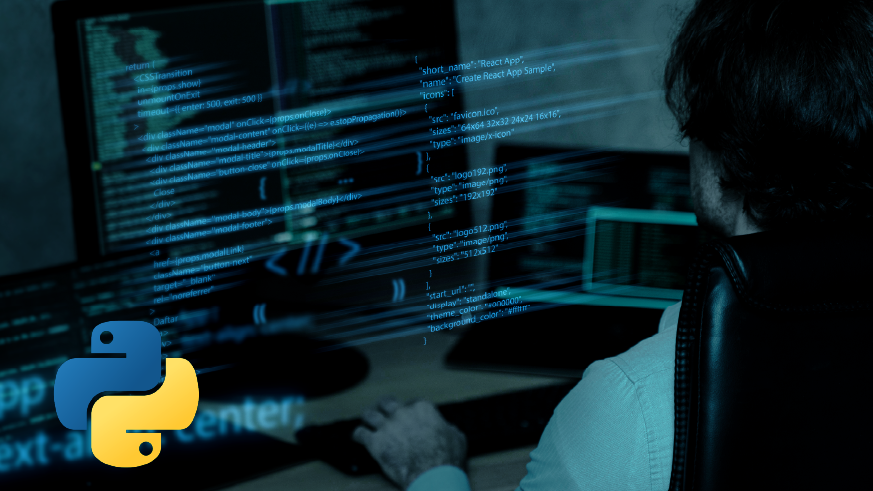
Introduction
Think about a world the place the kind of an object doesn’t matter so long as it performs the anticipated actions. This method makes your code extra versatile and simpler to handle. Enter duck typing—a robust programming mannequin that focuses on what an object can do quite than what it’s. This text will present you ways duck typing can remodel your use of object-oriented programming in Python, enhancing the readability and adaptability of your code.
Overview
- Perceive the idea of duck typing and its significance in Python programming.
- Discover ways to implement duck typing in Python with sensible examples.
- Establish the advantages of utilizing duck typing for versatile and maintainable code.
- Acknowledge potential pitfalls and greatest practices when utilizing duck typing.
- Apply duck typing to real-world Python tasks to enhance code adaptability.
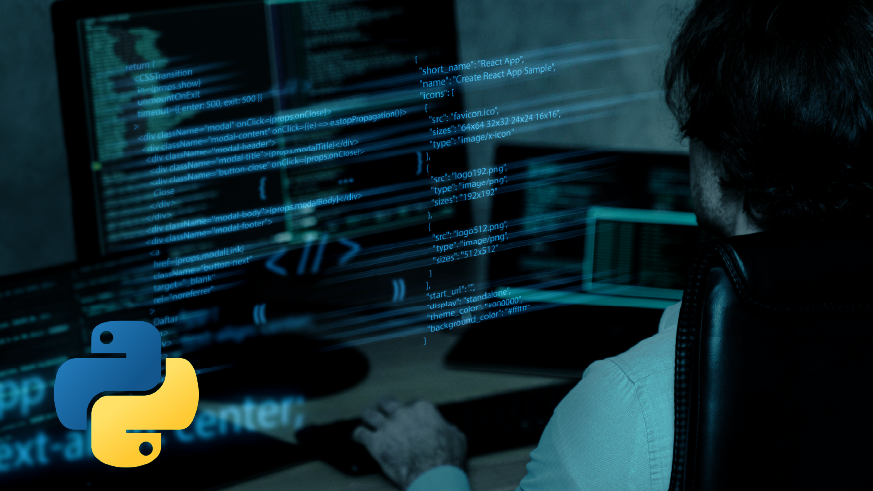
What’s Duck Typing?
An object suitability in a duck typing sort system shouldn’t be based mostly on sort of the thing however quite by the strategies and variables it possesses. Properly, in step with the saying ‘appears like a duck, swims like a duck, quacks like a duck, it’s a duck’, that is the place the time period comes from. This certainly implies that if an object performs the position of a selected sort, it may be utilized in Python because the stated sort.
Static Typing vs. Duck Typing
All variables and expressions in statically typed languages, similar to Java or C++, have their sorts identified at compile time. Whereas sort security is assured, this will additionally lead to a extra stiff and verbose code. As an example, in Java, a variable’s sort should be declared earlier than it could be used:
Checklist<String> record = new ArrayList<>();
record.add("Good day");
In distinction, Python makes use of dynamic typing, the place the kind of a variable is interpreted at runtime. Duck typing takes this a step additional by not checking the kind in any respect, however as a substitute checking for the presence of strategies or behaviors:
def add_to_list(obj, merchandise):
obj.append(merchandise)
my_list = [1, 2, 3]
add_to_list(my_list, 4)
Right here, add_to_list
will work with any object that has an append technique, not simply lists.
Advantages of Duck Typing
- Flexibility: You possibly can develop extra reusable and adaptable code by utilizing duck typing. So long as an object offers the mandatory strategies, you’ll be able to ship it to a perform.
- Simplicity: By disposing of sort declarations and specific interfaces, it makes programming easier.
- Polymorphism: Duck typing permits objects of various sorts for use interchangeably in the event that they implement the identical conduct, which promotes polymorphism.
- Ease of Refactoring: So long as the brand new object offers the identical strategies, you’ll be able to change an object’s sort with out altering the code that makes use of it, which facilitates refactoring.
Examples of Duck Typing
Let’s have a look at some sensible examples to grasp duck typing higher.
Instance 1: A Easy Operate
Take into consideration a perform that determines a form’s space. When utilizing duck typing, the perform merely must know that it has a approach to compute the realm—it doesn’t care what sort of form object it’s:
class Circle:
def __init__(self, radius):
self.radius = radius
def space(self):
return 3.14 * self.radius ** 2
class Sq.:
def __init__(self, aspect):
self.aspect = aspect
def space(self):
return self.aspect ** 2
def print_area(form):
print(f"The realm is {form.space()}")
circle = Circle(5)
sq. = Sq.(4)
print_area(circle)
print_area(sq.)
Output:
The realm is 78.5
The realm is 16
On this instance, print_area works with each Circle and Sq. objects as a result of they each have an space technique.
Instance 2: Collections and Iterators
Duck typing is especially helpful when working with collections and iterators. Suppose you wish to create a perform that prints all gadgets in a group:
def print_items(assortment):
for merchandise in assortment:
print(merchandise)
my_list = [1, 2, 3]
my_tuple = (4, 5, 6)
my_set = {7, 8, 9}
print_items(my_list)
print_items(my_tuple)
print_items(my_set)
Output:
1
2
3
4
5
6
7
8
9
The print_items perform works with lists, tuples, and units as a result of all of them help iteration.
Dealing with Errors with Duck Typing
One draw back of duck typing is that it may well result in runtime errors if an object doesn’t help the anticipated strategies. To deal with such instances, you need to use exception dealing with to catch errors gracefully:
def safe_append(obj, merchandise):
strive:
obj.append(merchandise)
besides AttributeError:
print(f"Object {obj} doesn't help the append technique")
my_list = [1, 2, 3]
my_string = "hi there"
safe_append(my_list, 4) # Works wonderful
safe_append(my_string, 'a') # Prints an error message
Output:
Object hi there doesn't help the append technique
Duck Typing in Observe
Duck typing is extensively utilized in Python libraries and frameworks. As an example, within the Python commonplace library, the json module makes use of duck typing to serialize objects to JSON:
import json
class CustomObject:
def to_json(self):
return {"title": "Customized", "worth": 42}
obj = CustomObject()
print(json.dumps(obj.to_json()))
Output:
{"title": "Customized", "worth": 42}
Right here, the json module expects objects to have a to_json technique to transform them to JSON-serializable codecs.
Conclusion
Python duck typing is an adaptable technique of object-oriented programming that prioritizes conduct above inheritance and kinds. This technique produces cleaner, extra maintainable code by enhancing the code’s intuitiveness and flexibility. It makes it potential to focus on an object’s capabilities, resulting in extra dependable and efficient programming strategies. Duck typing grows in usefulness as you’re employed with it and provides it to your Python toolbox.
Ceaselessly Requested Questions
A. Duck typing is a dynamic typing method the place an object’s suitability is decided by the presence of sure strategies and properties quite than the thing’s sort.
A. Static typing checks sorts at compile-time, whereas duck typing checks for technique and property presence at runtime, specializing in conduct quite than sort.
A. It’s based mostly on the saying, “If it appears like a duck and quacks like a duck, it should be a duck,” that means if an object behaves like a sure sort, it may be handled as that sort.