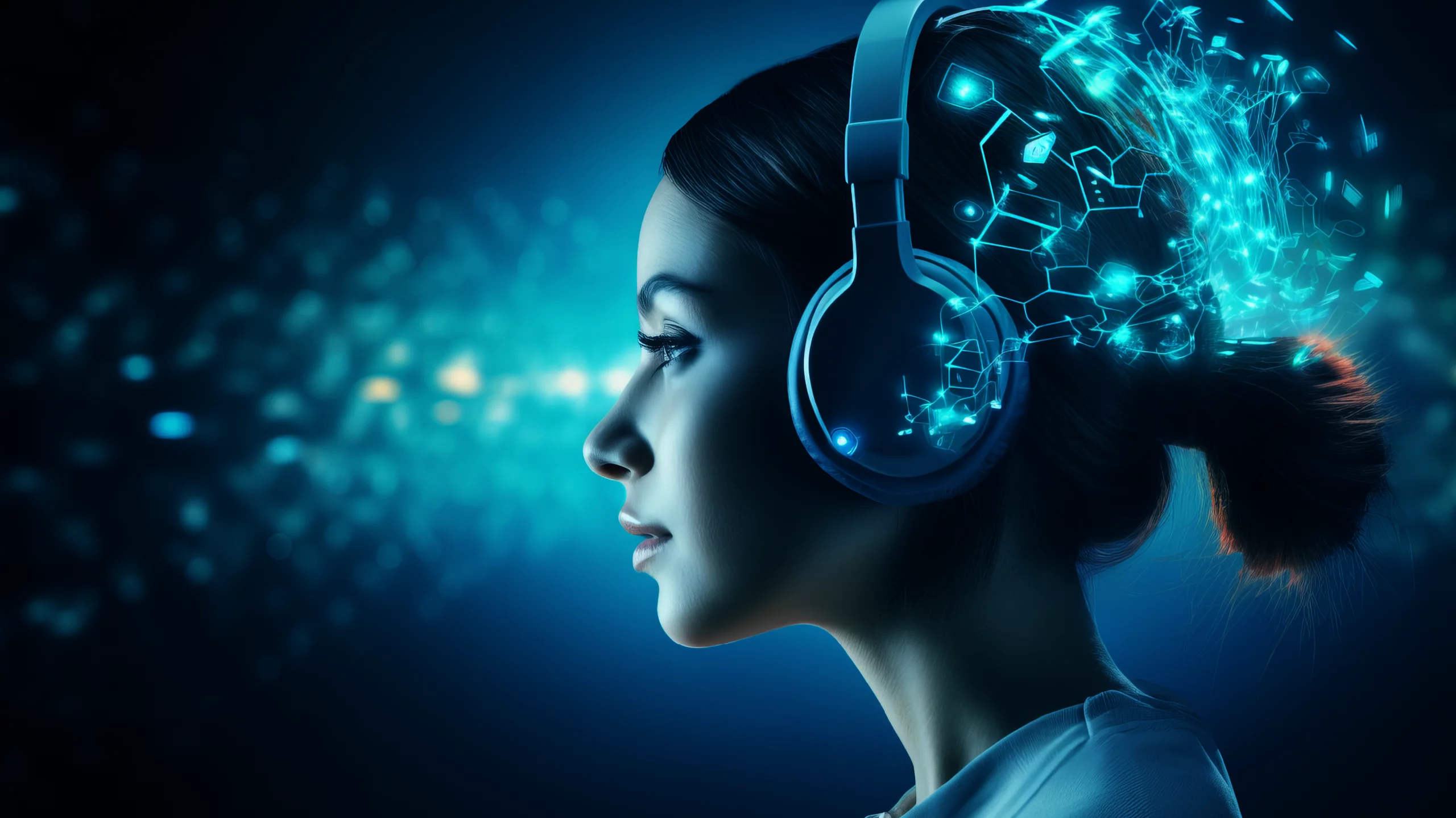
Introduction
Think about sifting by way of hundreds of images to seek out that one excellent shot—tedious, proper? Now, image a system that may do that in seconds, immediately presenting you with essentially the most related photos primarily based in your question. On this article, you’ll dive into the fascinating world of picture similarity search, the place we’ll remodel images into numerical vectors utilizing the highly effective VGG16 mannequin. With these vectors listed by FAISS, a instrument designed to swiftly and precisely find related objects, you’ll learn to construct a streamlined and environment friendly search system. By the tip, you’ll not solely grasp the magic behind vector embeddings and FAISS but additionally achieve hands-on expertise to implement your individual high-speed picture retrieval system.
Studying Goals
- Perceive how vector embeddings convert advanced knowledge into numerical representations for evaluation.
- Study the position of VGG16 in producing picture embeddings and its utility in picture similarity search.
- Acquire perception into FAISS and its capabilities for indexing and quick retrieval of comparable vectors.
- Develop expertise to implement a picture similarity search system utilizing VGG16 and FAISS.
- Discover challenges and options associated to high-dimensional knowledge and environment friendly similarity searches.
This text was revealed as part of the Knowledge Science Blogathon.
Understanding Vector Embeddings
Vector embeddings are a approach to characterize knowledge like photos, textual content, or audio as numerical vectors. On this illustration related objects are positioned close to one another in a high-dimensional area, which helps computer systems shortly discover and evaluate associated info.
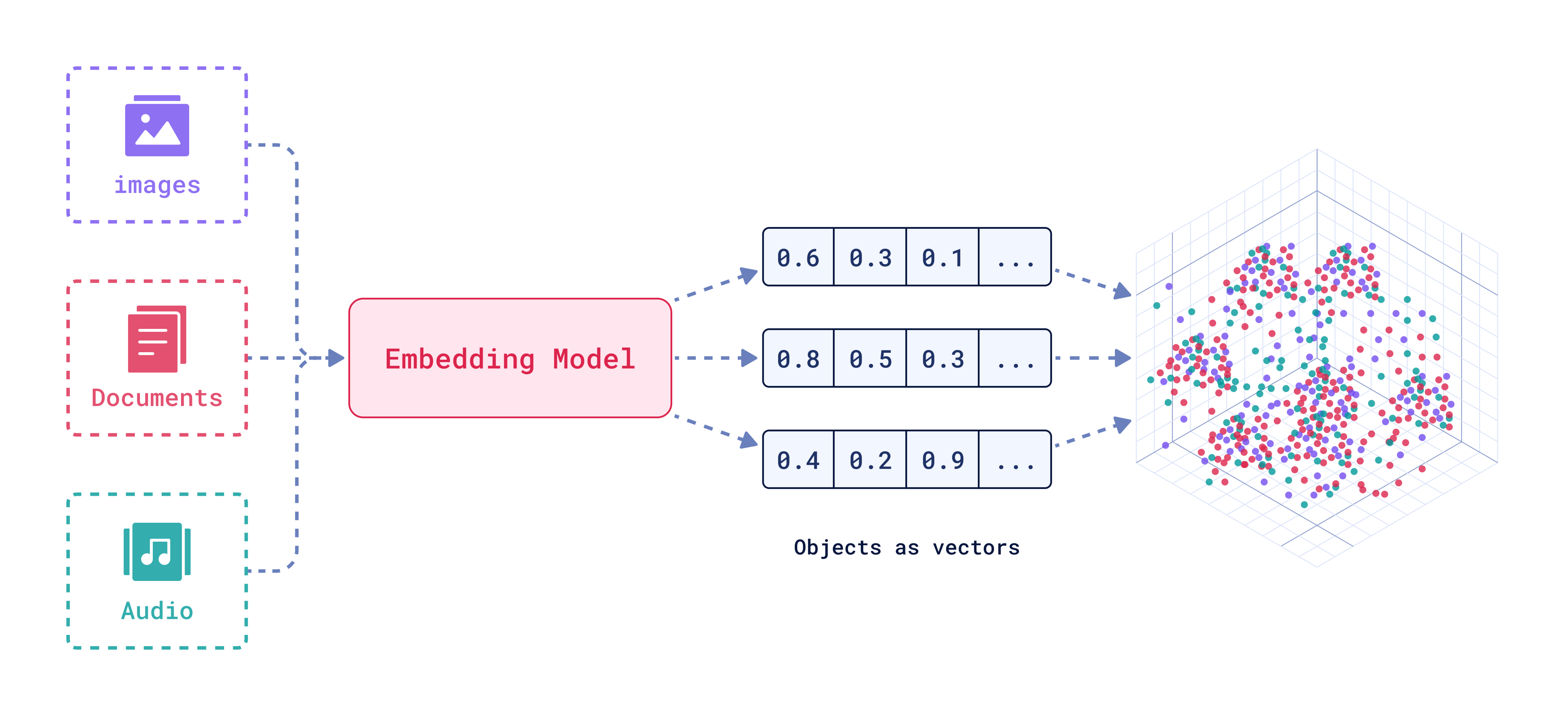
Benefits of Vector Embeddings
Allow us to now discover benefits of vector embeddings intimately.
- Vector embeddings are time environment friendly as distance between vectors may be computed quickly.
- Embeddings deal with massive dataset effectively making them scalable and appropriate for large knowledge functions.
- Excessive-dimensional knowledge, comparable to photos, can characterize lower-dimensional areas with out shedding vital info. This illustration simplifies storage and enhances area effectivity. It captures the semantic which means between knowledge objects, resulting in extra correct ends in duties requiring contextual understanding, comparable to NLP and picture recognition.
- Vector embeddings are versatile as they are often utilized to totally different knowledge varieties
- Pre-trained embeddings and vector databases can be found which cut back the necessity for intensive coaching of information thus we save on computational sources.
- Historically function engineering methods require guide creation and choice of options, embeddings automate function engineering course of by studying options from knowledge.
- Embeddings are extra adaptable to new inputs higher as in comparison with rule-based fashions.
- Graph primarily based approaches additionally seize advanced relations however require extra advanced knowledge buildings and algorithms, embeddings are computationally much less intensive.
What’s VGG16?
We can be utilizing VGG16 to calculate our picture embeddings .VGG16 is a Convolutional Neural Community it’s an object detection and classification algorithm. The 16 stands for the 16 layers with learnable weights within the community.
Beginning with an enter picture and resizing it to 224×224 pixels with three shade channels . These at the moment are handed to a convolutional layers that are like a collection of filters that take a look at small elements of the picture. Every filter right here captures totally different options comparable to edges, colours, textures and so forth. VGG16 makes use of 3X3 filters which signifies that they take a look at 3X3 pixel space at a time. There are 13 convolutional layers in VGG16.That is adopted by the Activation Perform (ReLU) which stands for Rectified Linear Unit and it provides non linearity to the mannequin which permits it to be taught extra advanced patters.
Subsequent are the Pooling Layers which cut back the dimensions of the picture illustration by taking an important info from small patches which shrinks the picture whereas protecting the necessary options.VGG16 makes use of 2X2 pooling layers which implies it reduces the picture measurement by half. Lastly the Absolutely Linked Layer receives the output and makes use of all the knowledge that the convolutional layers have discovered to reach at a closing conclusion. The output layer determines which class the enter picture most probably belongs to by producing possibilities for every class utilizing a softmax operate.

First load the mannequin then use VGG16 with pre-trained weights however take away the final layer that offers the ultimate classification. Now resize and preprocess the pictures to suit the mannequin’s necessities which is 224 X 224 pixels and eventually compute embeddings by passing the pictures by way of the mannequin from one of many absolutely linked layers.
Utilizing FAISS for Indexing
Fb AI Analysis developed Fb AI Similarity Search (FAISS) to effectively search and cluster dense vectors. FAISS excels at dealing with large-scale datasets and shortly discovering objects much like a question.
What’s Similarity Looking out ?
FAISS constructs an index in RAM while you present a vector of dimension (d_i). This index, an object with an add
technique, shops the (x_i) vector, assuming a hard and fast dimension for (x_i).
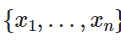
After the construction is constructed when a brand new vector x is supplied in dimension d it performs the next operation. Computing the argmin is the search operation on the index.

FAISS discover the objects within the index closest to the brand new merchandise.
Right here || .|| represents the Euclidean distance (L2). The closeness is measured utilizing Euclidean distance.
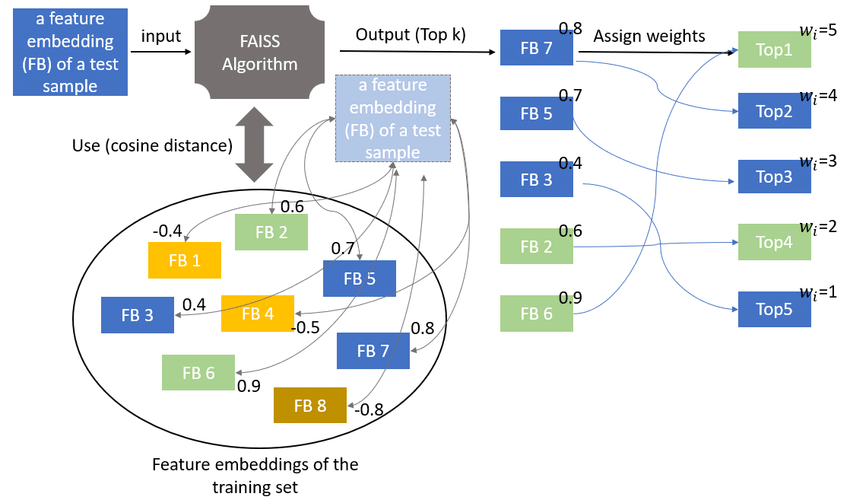
Code Implementation utilizing Vector Embeddings
We are going to now look into code Implementation for detection of comparable photos utilizing vector embeddings.
Step 1: Import Libraries
Import obligatory libraries for picture processing, mannequin dealing with, and similarity looking.
import cv2
import numpy as np
import faiss
import os
from keras.functions.vgg16 import VGG16, preprocess_input
from keras.preprocessing import picture
from keras.fashions import Mannequin
from google.colab.patches import cv2_imshow
Step 2: Load Photographs from Folder
Outline a operate to load all photos from a specified folder and its subfolders.
# Load photos from folder and subfolders
def load_images_from_folder(folder):
photos = []
image_paths = []
for root, dirs, recordsdata in os.stroll(folder):
for file in recordsdata:
if file.endswith(('jpg', 'jpeg', 'png')):
img_path = os.path.be a part of(root, file)
img = cv2.imread(img_path)
if img shouldn't be None:
photos.append(img)
image_paths.append(img_path)
return photos, image_paths
Step 3: Load Pre-trained Mannequin and Take away Prime Layers
Load the VGG16 mannequin pre-trained on ImageNet and modify it to output embeddings from the ‘fc1’ layer.
# Load pre-trained mannequin and take away high layers
base_model = VGG16(weights="imagenet")
mannequin = Mannequin(inputs=base_model.enter, outputs=base_model.get_layer('fc1').output)
Step 4: Compute Embeddings Utilizing VGG16
Outline a operate to compute picture embeddings utilizing the modified VGG16 mannequin.
# Compute embeddings utilizing VGG16
def compute_embeddings(photos)
embeddings = []
for img in photos:
img = cv2.resize(img, (224, 224))
img = picture.img_to_array(img)
img = np.expand_dims(img, axis=0)
img = preprocess_input(img)
img_embedding = mannequin.predict(img)
embeddings.append(img_embedding.flatten())
return np.array(embeddings)
Step 5: Create FAISS Index
Outline a operate to create a FAISS index from the computed embeddings.
def create_index(embeddings):
d = embeddings.form[1]
index = faiss.IndexFlatL2(d)
index.add(embeddings)
return index
Step 6: Load Photographs and Compute Embeddings
Load photos, compute their embeddings, and create a FAISS index.
photos, image_paths = load_images_from_folder('photos')
embeddings = compute_embeddings(photos)
index = create_index(embeddings)
Step 7: Seek for Comparable Photographs
Outline a operate to seek for essentially the most related photos within the FAISS index.
def search_similar_images(index, query_embedding, top_k=1):
D, I = index.search(query_embedding, top_k)
return I
Step 8: Instance Utilization
Load a question picture, compute its embedding, and seek for related photos.
# Seek for related photos
def search_similar_images(index, query_embedding, top_k=1):
D, I = index.search(query_embedding, top_k)
return I
Step 9: Show Outcomes
Print the indices and file paths of the same photos.
print("Comparable photos indices:", similar_images_indices)
for idx in similar_images_indices[0]:
print(image_paths[idx])
Step 10: Show Photographs Utilizing cv2_imshow
Show the question picture and essentially the most related photos utilizing OpenCV’s cv2_imshow
.
print("Question Picture")
cv2_imshow(query_image)
cv2.waitKey(0) # Anticipate a key press to shut the picture
for idx in similar_images_indices[0]:
similar_image = cv2.imread(image_paths[idx])
print("Most Comparable Picture")
cv2_imshow(similar_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
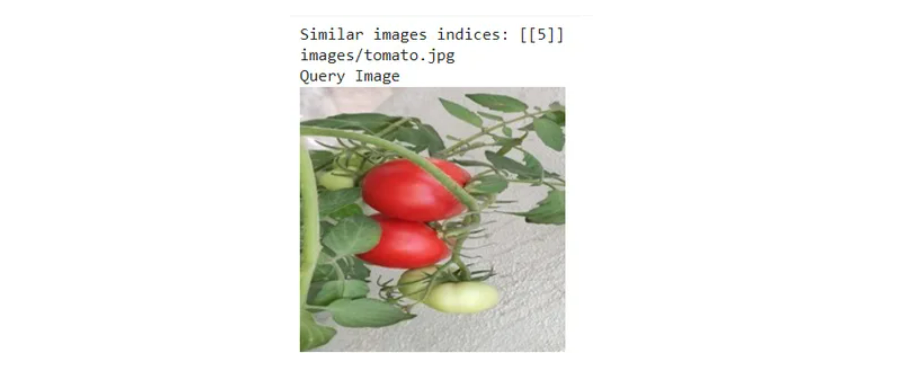
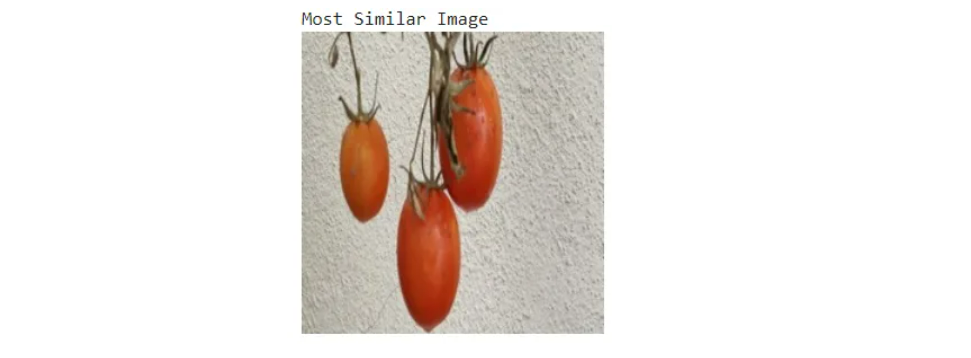
I’ve made use of Vegetable photos dataset for code implementation the same dataset may be discovered right here.
Challenges Confronted
- Storing excessive dimensional embeddings for a lot of photos consumes a considerable amount of reminiscence.
- Producing embeddings and performing similarity searches may be computationally intensive
- Variations in picture high quality, measurement and format can have an effect on the accuracy of embeddings.
- Creating and updating the FAISS index may be time-consuming incase of very massive datasets.
Conclusion
We explored the creation of a picture similarity search system by leveraging vector embeddings and FAISS (Fb AI Similarity Search). We started by understanding vector embeddings and their position in representing photos as numerical vectors, facilitating environment friendly similarity searches. Utilizing the VGG16 mannequin, we computed picture embeddings by processing and resizing photos to extract significant options. We then created a FAISS index to effectively handle and search by way of these embeddings.
Lastly, we demonstrated the best way to question this index to seek out related photos, highlighting the benefits and challenges of working with high-dimensional knowledge and similarity search methods. This strategy underscores the ability of mixing deep studying fashions with superior indexing strategies to boost picture retrieval and comparability capabilities.
Key Takeaways
- Vector embeddings remodel advanced knowledge like photos into numerical vectors for environment friendly similarity searches.
- VGG16 mannequin extracts significant options from photos, creating detailed embeddings for comparability.
- FAISS indexing accelerates similarity searches by effectively managing and querying massive units of picture embeddings.
- Picture similarity search leverages superior indexing methods to shortly establish and retrieve comparable photos.
- Dealing with high-dimensional knowledge entails challenges in reminiscence utilization and computational sources however is essential for efficient similarity searches.
Often Requested Questions
A. Vector embeddings are numerical representations of information components, comparable to photos, textual content, or audio. They place related objects shut to one another in a high-dimensional area, enabling environment friendly similarity searches and comparisons by computer systems.
A. Vector embeddings simplify and speed up discovering and evaluating related objects in massive datasets. They permit for environment friendly computation of distances between knowledge factors, making them excellent for duties requiring fast similarity searches and contextual understanding.
A. FAISS, developed by Fb AI Analysis, effectively searches and clusters dense vectors. Designed for large-scale datasets, it shortly retrieves related objects by creating and looking by way of an index of vector embeddings.
A. FAISS operates by creating an index from the vector embeddings of your knowledge. If you provide a brand new vector, FAISS searches the index to seek out the closest vectors. It usually measures similarity utilizing Euclidean distance (L2).
The media proven on this article shouldn’t be owned by Analytics Vidhya and is used on the Writer’s discretion.