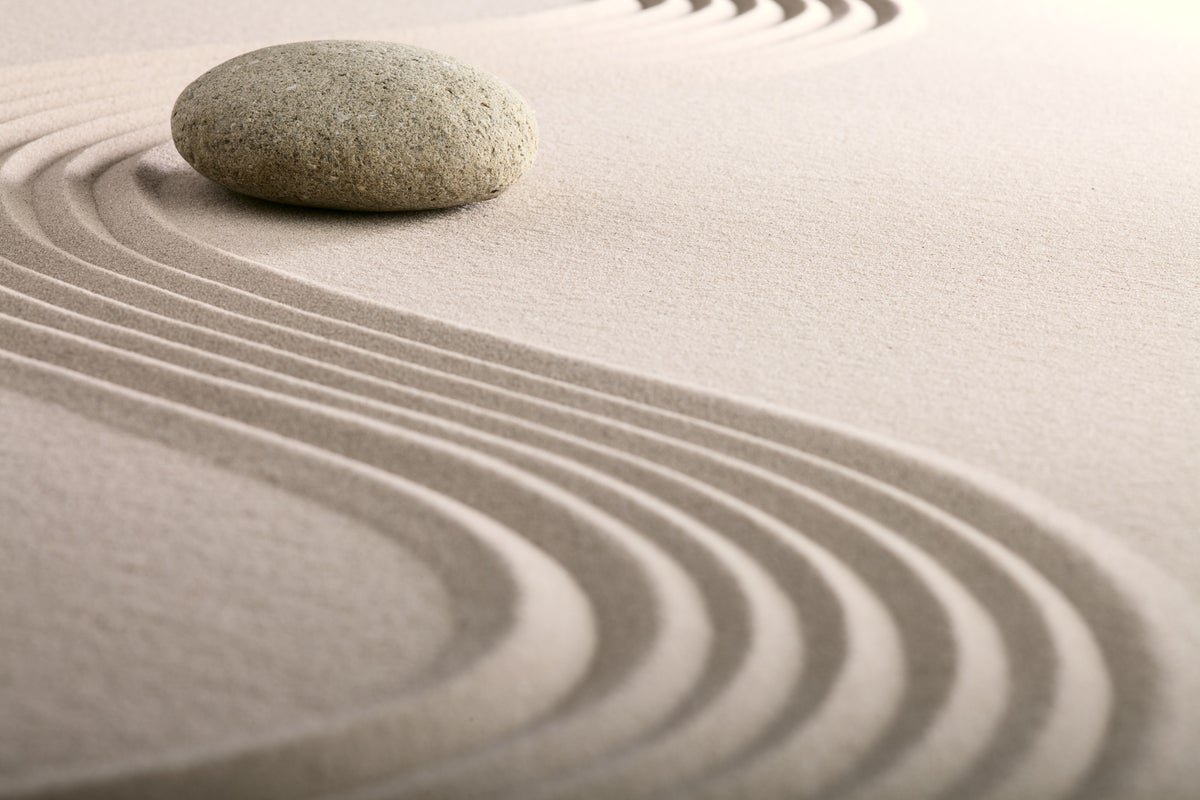
The JavaScript language is likely one of the wonders of the software program world. It’s extremely highly effective, versatile, and versatile. One limitation of its elementary design, nevertheless, is its single-threaded nature. Conventional JavaScript seems to deal with parallel duties, however that may be a trick of syntax. To attain true parallelism, it’s worthwhile to use trendy multithreading approaches like net staff and employee threads.
Parallelism vs. concurrency
Probably the most primary solution to perceive the distinction between parallelism and concurrency is that concurrency is semantic whereas parallelism is implementation. What I imply is that concurrency enables you to inform the system (semantics) to do multiple factor directly. Parallelism merely performs a number of duties concurrently (implementation). All parallel processing is concurrent, however not all concurrent programming is parallel.
In vanilla JavaScript, you may inform the platform to do a few issues:
perform fetchPerson(id) {
return new Promise((resolve, reject) => {
fetch(`https://swapi.dev/api/individuals/${id}`)
.then(response => response.json())
.then(knowledge => resolve(knowledge))
.catch(error => reject(error));
});
}
const lukeId = 1;
const leiaId = 5;
console.log("Fetching Star Wars characters...");
// Fetch character knowledge concurrently (non-blocking)
Promise.all([fetchPerson(lukeId), fetchPerson(leiaId)])
.then(knowledge => {
console.log("Characters obtained:");
console.log(knowledge[0]); // Knowledge for Luke Skywalker (ID: 1)
console.log(knowledge[1]); // Knowledge for Leia Organa (ID: 5)
})
.catch(error => console.error("Error fetching characters:", error));
console.log("Shifting on to different issues...");
// Fetching Star Wars characters...
// Shifting on to different issues...
Characters obtained:
{identify: 'Luke Skywalker', peak: '172', mass: '77', …}
{identify: 'Leia Organa', peak: '150', mass: '49', …}
This seems to fetch knowledge on Luke and Leia on the similar time, through the use of Promise.all
to execute two fetch
calls collectively. In reality, although, JavaScript will schedule every process to be dealt with by the one utility thread.
It is because JavaScript makes use of an occasion loop. The loop picks stuff off of a queue so quick that it usually seems to occur concurrently—but it surely’s not a very concurrent course of.
To actually do two issues directly, we’d like a number of threads. Threads are an abstraction of the underlying working system’s processes and their entry to the {hardware}, together with multi-core processors.
Multithreading with net staff
Internet staff provide you with a solution to spawn threads in an online browser. You possibly can simply load a separate employee script from the primary script, and it’ll deal with asynchronous messages. Every message handler is run in its personal thread, providing you with true parallelism.
For our easy Star Wars API instance, we need to spawn threads that can deal with or fetch requests. Utilizing net staff for that is overkill, clearly, but it surely retains issues easy. We need to create an online employee that can settle for a message from the primary thread and concern the requests.
Right here’s what our essential script (essential.js
) appears to be like like now:
perform fetchPersonWithWorker(id) {
return new Promise((resolve, reject) => {
const employee = new Employee('employee.js');
employee.onmessage = perform(occasion) {
if (occasion.knowledge.error) {
reject(occasion.knowledge.error);
} else {
resolve(occasion.knowledge);
}
employee.terminate(); // Clear up the employee after receiving the information
}
employee.postMessage({ url: `https://swapi.dev/api/individuals/${id}` });
});
}
const lukeId = 1; const leiaId = 5;
console.log("Fetching Star Wars characters with net employee...");
// Fetch character knowledge concurrently (really parallel)
Promise.all([fetchPersonWithWorker(lukeId), fetchPersonWithWorker(leiaId)])
.then(knowledge => {
console.log("Characters obtained:");
console.log(knowledge[0]); // Knowledge for Luke Skywalker (ID: 1)
console.log(knowledge[1]); // Knowledge for Leia Organa (ID: 5)
})
.catch(error => console.error("Error fetching characters:", error));
console.log("Shifting on to different issues...");
That is just like the primary instance, however as an alternative of utilizing a perform that works regionally in Promise.all
, we cross within the fetchPersonWithWorker
perform. This latter perform creates a Employee
object known as employee
, which is configured with the employee.js
file.
As soon as the employee object is created, we offer an onmessage
occasion on it. We are going to use this to deal with the messages getting back from the employee. In our case, we resolve or reject the promise we’re returning (consumed by Promise.all
in the primary script), then we terminate the employee.
After that, we name employee.postMessage()
and cross in a easy JSON object with a URL subject set to the URL we need to name.
The net employee
Right here’s the opposite aspect of the equation, in employee.js
:
// employee.js
onmessage = perform(occasion) {
console.log(“onmessage: “ + occasion.knowledge); // {"url":"https://swapi.dev/api/individuals/1"}
const { url } = occasion.knowledge;
fetch(url)
.then(response => response.json())
.then(knowledge => postMessage(knowledge))
.catch(error => postMessage({ error }));
}
Our easy onmessage
handler accepts the occasion and makes use of the URL subject to concern the identical fetch calls as earlier than, however this time we use postMessage()
to speak the outcomes again to essential.js
.
So, you may see we talk between the 2 worlds with messages utilizing postMessage
and onmessage
. Keep in mind: the onmessage
handlers within the employee happen asynchronously in their very own threads. (Don’t use native variables to retailer knowledge—they’re prone to be wiped away).
Server-side threading with employee threads
Now let’s check out the server aspect, utilizing Node.js. On this case, as an alternative of net staff, we use the idea of a employee thread. A employee thread is just like an online employee in that we cross messages forwards and backwards from the primary thread to the employee.
For instance, as an instance now we have two recordsdata, essential.js
and employee.js
. We’ll run essential.js
(utilizing the command: node essential.js
) and it’ll spawn a thread by loading employee.js
as a employee thread. Right here is our essential.js
file:
const { Employee } = require('worker_threads');
perform fetchPersonWithWorker(id) {
return new Promise((resolve, reject) => {
const employee = new Employee('./employee.js', { workerData: id });
employee.on('message', (knowledge) => {
if (knowledge.error) {
reject(knowledge.error);
} else {
resolve(knowledge);
}
employee.terminate();
});
employee.on('error', (error) => reject(error));
let url = `https://swapi.dev/api/individuals/${id}`;
employee.postMessage({ url });
});
}
const lukeId = 1;
const leiaId = 5;
console.log("Fetching Star Wars characters with employee threads...");
Promise.all([fetchPersonWithWorker(lukeId), fetchPersonWithWorker(leiaId)])
.then(knowledge => {
console.log("Characters obtained: "+ JSON.stringify(knowledge) );
console.log(knowledge[0]); // Knowledge for Luke Skywalker (ID: 1)
console.log(knowledge[1]); // Knowledge for Leia Organa (ID: 5)
})
.catch(error => console.error("Error fetching characters:", error));
console.log("Shifting on to different issues...");
We import Employee
from the worker_threads
module, however be aware that it is constructed into Node, so we don’t want NPM for this. To launch the employee, we create a brand new Employee
object and provides it the employee.js
file as a parameter. As soon as that’s achieved, we add a message listener that resolves or rejects our promise—that is precisely like we did for the online employee. We additionally terminate the employee when achieved, to wash up the sources.
Lastly, we ship the employee a brand new message containing the URL we need to retrieve.
The employee thread
Right here’s a have a look at employee.js:
const { parentPort } = require('worker_threads');
parentPort.on('message', (msg) => {
console.log("message(employee): " + msg.url);
fetch(msg.url)
.then(response => response.json())
.then(knowledge => parentPort.postMessage(knowledge))
.catch(error => parentPort.postMessage({ error }));
});
Once more we import from worker_threads
, this time the parentPort
object. That is an object that permits us to speak with the primary thread. In our case, we pay attention for the message occasion, and when it’s obtained, we unpack the url subject from it and use that to concern a request.
On this manner now we have achieved really concurrent requests to the URLs. In case you run the pattern with node essential.js
, you’ll see the information from each URLs output to the console.
Conclusion
You’ve got seen the basic mechanisms for reaching really parallel threads in JavaScript, each within the browser and on the server. How these are run depends upon the working system and {hardware} profile of the particular host setting, however basically, they offer you entry to multithreaded processes.
Whereas JavaScript doesn’t help the vary and depth of concurrent programming present in a language like Java, net staff and employee threads get you the fundamental mechanism for parallelism if you want it.
You’ll find the runnable samples for this text on GitHub right here. To run the online employee instance, kind
$ node server.js
from the foundation listing.
To run the employee thread instance, kind:
~/worker-thread $ node essential.js
Copyright © 2024 IDG Communications, Inc.