Introduction
Computing sq. root is a important idea in arithmetic and inside this programming language one is ready to embark on this fundamental computation in a quite simple and environment friendly method. Whether or not you’re concerned in an experiment, simulations, knowledge evaluation or utilizing machine studying, calculating sq. roots in Python is essential. On this information, you’ll be taught varied methods of approximating sq. root in Python; whether or not it’s by use of inbuilt capabilities or by utilizing different developed python libraries that are environment friendly in fixing arithmetic computation.
Studying Outcomes
- Perceive what a sq. root is and its significance in programming.
- Learn to compute sq. roots utilizing Python’s built-in
math
module. - Uncover other ways to search out sq. roots utilizing exterior libraries like
numpy
. - Be capable to deal with edge instances similar to adverse numbers.
- Implement sq. root calculations in real-world examples.
What’s a Sq. Root?
A sq. root of a quantity is a worth that, when multiplied by itself, ends in the unique quantity. Mathematically, if y is the sq. root of x, then:

This implies 𝑦 × 𝑦 = 𝑥. For instance, the sq. root of 9 is 3 as a result of 3 × 3 = 9.
Notation:
The sq. root of a quantity xxx is often denoted as:
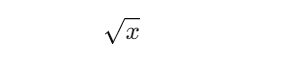
Why It’s Vital to Perceive Sq. Roots
Sq. roots are important throughout arithmetic, sciences, and technical professions as a result of they floor understanding of those operations. Whether or not you might be simply doing addition and subtraction, or fixing equations that contain troublesome algorithms, the understanding of how sq. roots work can allow you resolve many issues successfully. Beneath are a number of key explanation why understanding sq. roots is necessary:
- Basis for Algebra: Sq. roots are important for fixing quadratic equations and understanding powers and exponents.
- Geometry and Distance: Sq. roots assist calculate distances, areas, and diagonals, particularly in geometry and structure.
- Physics and Engineering: Key formulation in physics and engineering, like velocity and stress evaluation, contain sq. roots.
- Monetary Calculations: Utilized in danger evaluation, customary deviation, and development fashions in finance and economics.
- Information Science & Machine Studying: Sq. roots are very important in optimization algorithms, error measurements, and statistical capabilities.
- Constructing Downside-Fixing Abilities: Enhances mathematical reasoning and logic for tackling extra complicated issues.
Strategies to Compute Sq. Roots in Python
Python being an open supply language, there are a lot of methods to reach on the sq. root of a quantity relying on the conditions at hand. Beneath are the commonest strategies, together with detailed descriptions:
Utilizing the math.sqrt()
Perform
The straightforward and customary technique for locating the sq. root of a floating quantity in Python makes use of the maths.sqrt() operate from the inbuilt math library.
Instance:
import math
print(math.sqrt(25)) # Output: 5.0
print(math.sqrt(2)) # Output: 1.4142135623730951
The math.sqrt()
operate solely works with non-negative numbers and returns a float. If a adverse quantity is handed, it raises a ValueError
.
Utilizing the cmath.sqrt()
Perform for Advanced Numbers
For instances the place you could compute the sq. root of adverse numbers, the cmath.sqrt()
operate from the cmath
(complicated math) module is used. This technique returns a fancy quantity consequently.
This technique permits the computation of sq. roots of each optimistic and adverse numbers. It returns a fancy quantity (within the type of a + bj
) even when the enter is adverse.
Instance:
import cmath
print(cmath.sqrt(-16)) # Output: 4j
print(cmath.sqrt(25)) # Output: (5+0j)
It’s used when working with complicated numbers or when adverse sq. roots should be calculated.
Utilizing the Exponentiation Operator **
In Python, the exponentiation operator (**
) can be utilized to calculate sq. roots by elevating a quantity to the ability of 1/2 (0.5).
This technique works for each integers and floats. If the quantity is optimistic, it returns a float. If the quantity is adverse, it raises a ValueError
, as this technique doesn’t deal with complicated numbers.
Instance:
print(16 ** 0.5) # Output: 4.0
print(2 ** 0.5) # Output: 1.4142135623730951
A fast and versatile technique that doesn’t require importing any modules, appropriate for easy calculations.
Utilizing Newton’s Methodology (Iterative Approximation)
Newton and its different identify the Babylonian technique is an easy algorithm to estimate the sq. root of a given amount. This technique is as follows and isn’t as simple because the built-in capabilities: Nonetheless, by doing this, one will get to know how sq. roots are computed within the background.
This one merely begins with some guess, often center worth, after which iteratively refines this guess till the specified precision is attained.
Instance:
def newtons_sqrt(n, precision=0.00001):
guess = n / 2.0
whereas abs(guess * guess - n) > precision:
guess = (guess + n / guess) / 2
return guess
print(newtons_sqrt(16)) # Output: roughly 4.0
print(newtons_sqrt(2)) # Output: roughly 1.41421356237
Helpful for customized precision necessities or academic functions to show the approximation of sq. roots.
Utilizing the pow()
Perform
Python additionally has a built-in pow()
operate, which can be utilized to calculate the sq. root by elevating the quantity to the ability of 0.5.
Instance:
num = 25
end result = pow(num, 0.5)
print(f'The sq. root of {num} is {end result}')
#Output: The sq. root of 25 is 5.0
Utilizing numpy.sqrt()
for Arrays
If you’re doing operations with arrays or matrices, within the numpy library there’s the numpy.sqrt() operate which is optimized for such calculations as acquiring sq. root of each component within the array.
One of many advantages of utilizing this strategy is that to search out sq. root of matching component you don’t recompute the entire trigonometric chain, so is appropriate for giant knowledge units.
Instance:
import numpy as np
arr = np.array([4, 9, 16, 25])
print(np.sqrt(arr)) # Output: [2. 3. 4. 5.]
Splendid for scientific computing, knowledge evaluation, and machine studying duties the place operations on massive arrays or matrices are frequent.
Comparability of Strategies
Beneath is the desk of evaluating the strategies of python sq. root strategies:
Methodology | Handles Destructive Numbers | Handles Advanced Numbers | Splendid for Arrays | Customizable Precision |
---|---|---|---|---|
math.sqrt() |
No | No | No | No |
cmath.sqrt() |
Sure | Sure | No | No |
Exponentiation (** ) |
No | No | No | No |
Newton’s Methodology | No (with out complicated dealing with) | No (with out complicated dealing with) | No | Sure (with customized implementation) |
numpy.sqrt() |
No | Sure | Sure | No |
Actual-World Use Instances for Sq. Roots
- In Information Science: Clarify how sq. roots are used to calculate customary deviation, variance, and root imply sq. errors in machine studying fashions.
import numpy as np
knowledge = [2, 4, 4, 4, 5, 5, 7, 9]
standard_deviation = np.std(knowledge)
print(f"Customary Deviation: {standard_deviation}")
- In Graphics & Animation: Sq. roots are generally utilized in computing distances between factors (Euclidean distance) in 2D or 3D areas.
point1 = (1, 2)
point2 = (4, 6)
distance = math.sqrt((point2[0] - point1[0])**2 + (point2[1] - point1[1])**2)
print(f"Distance: {distance}") # Output: 5.0
- Visualization: We will use python sq. root for visualizing the info.
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sqrt(x)
plt.plot(x, y)
plt.title("Sq. Root Perform")
plt.xlabel("x")
plt.ylabel("sqrt(x)")
plt.present()
Efficiency Issues and Optimization
Add a bit evaluating the computational effectivity of various sq. root strategies, particularly when working with massive datasets or real-time methods.
Instance:
import timeit
print(timeit.timeit("math.sqrt(144)", setup="import math"))
print(timeit.timeit("144 ** 0.5"))
This may spotlight the professionals and cons of utilizing one technique over one other, particularly by way of execution velocity and reminiscence consumption.
Dealing with Edge Instances
Dealing with edge instances is essential when working with sq. root calculations in Python to make sure robustness and accuracy. This part will discover handle particular instances like adverse numbers and invalid inputs successfully.
- Error Dealing with: Focus on deal with exceptions when calculating sq. roots, similar to utilizing
try-except
blocks to handleValueError
for adverse numbers inmath.sqrt()
.
attempt:
end result = math.sqrt(-25)
besides ValueError:
print("Can't compute the sq. root of a adverse quantity utilizing math.sqrt()")
- Advanced Roots: Present extra detailed examples for dealing with complicated roots utilizing
cmath.sqrt()
and clarify when such instances happen, e.g., in sign processing or electrical engineering.
Conclusion
Python presents a number of decisions in relation to utilizing for sq. roots; the essential kind is math.sqrt() whereas the extra developed kind is numpy.sqrt() for arrays. Thus, you may choose the mandatory technique to make use of for a specific case. Moreover, information of use cmath to unravel particular instances like the usage of adverse numbers will assist to make your code considerable.
Incessantly Requested Questions
A. The best method is to make use of the math.sqrt()
operate, which is easy and efficient for many instances.
A. But there’s a cmath.sqrt() operate which takes complicated quantity and turns into imaginary for ratio lower than zero.
A. Sure, by utilizing the numpy.sqrt()
operate, you may calculate the sq. root for every component in an array or record.
A. Passing a adverse quantity to math.sqrt()
will elevate a ValueError
as a result of sq. roots of adverse numbers should not actual.
pow(x, 0.5)
the identical as math.sqrt(x)
?
A. Sure, pow(x, 0.5)
is mathematically equal to math.sqrt(x)
, and each return the identical end result for non-negative numbers.